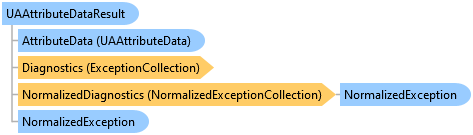
'Declaration
<CLSCompliantAttribute(True)> <ComDefaultInterfaceAttribute(OpcLabs.EasyOpc.UA.OperationModel.ComTypes._UAAttributeDataResult)> <ComVisibleAttribute(True)> <GuidAttribute("111C8C94-4928-4A45-8127-CC73667A2428")> <TypeConverterAttribute(System.ComponentModel.ExpandableObjectConverter)> <ValueControlAttribute("OpcLabs.BaseLib.Forms.Common.ObjectSerializationControl, OpcLabs.BaseLibForms, Version=5.81.455.1, Culture=neutral, PublicKeyToken=6faddca41dacb409", DefaultReadWrite=False, Export=True, PageId=10001)> <SerializableAttribute()> Public Class UAAttributeDataResult Inherits OpcLabs.BaseLib.OperationModel.OperationResult Implements LINQPad.ICustomMemberProvider, OpcLabs.BaseLib.ComTypes._Info, OpcLabs.BaseLib.ComTypes._Object2, OpcLabs.BaseLib.OperationModel.ComTypes._OperationResult, OpcLabs.EasyOpc.UA.OperationModel.ComTypes._UAAttributeDataResult, System.ICloneable, System.IFormattable, System.Runtime.Serialization.ISerializable, System.Xml.Serialization.IXmlSerializable
'Usage
Dim instance As UAAttributeDataResult
[CLSCompliant(true)] [ComDefaultInterface(OpcLabs.EasyOpc.UA.OperationModel.ComTypes._UAAttributeDataResult)] [ComVisible(true)] [Guid("111C8C94-4928-4A45-8127-CC73667A2428")] [TypeConverter(System.ComponentModel.ExpandableObjectConverter)] [ValueControl("OpcLabs.BaseLib.Forms.Common.ObjectSerializationControl, OpcLabs.BaseLibForms, Version=5.81.455.1, Culture=neutral, PublicKeyToken=6faddca41dacb409", DefaultReadWrite=false, Export=true, PageId=10001)] [Serializable()] public class UAAttributeDataResult : OpcLabs.BaseLib.OperationModel.OperationResult, LINQPad.ICustomMemberProvider, OpcLabs.BaseLib.ComTypes._Info, OpcLabs.BaseLib.ComTypes._Object2, OpcLabs.BaseLib.OperationModel.ComTypes._OperationResult, OpcLabs.EasyOpc.UA.OperationModel.ComTypes._UAAttributeDataResult, System.ICloneable, System.IFormattable, System.Runtime.Serialization.ISerializable, System.Xml.Serialization.IXmlSerializable
[CLSCompliant(true)] [ComDefaultInterface(OpcLabs.EasyOpc.UA.OperationModel.ComTypes._UAAttributeDataResult)] [ComVisible(true)] [Guid("111C8C94-4928-4A45-8127-CC73667A2428")] [TypeConverter(System.ComponentModel.ExpandableObjectConverter)] [ValueControl("OpcLabs.BaseLib.Forms.Common.ObjectSerializationControl, OpcLabs.BaseLibForms, Version=5.81.455.1, Culture=neutral, PublicKeyToken=6faddca41dacb409", DefaultReadWrite=false, Export=true, PageId=10001)] [Serializable()] public ref class UAAttributeDataResult : public OpcLabs.BaseLib.OperationModel.OperationResult, LINQPad.ICustomMemberProvider, OpcLabs.BaseLib.ComTypes._Info, OpcLabs.BaseLib.ComTypes._Object2, OpcLabs.BaseLib.OperationModel.ComTypes._OperationResult, OpcLabs.EasyOpc.UA.OperationModel.ComTypes._UAAttributeDataResult, System.ICloneable, System.IFormattable, System.Runtime.Serialization.ISerializable, System.Xml.Serialization.IXmlSerializable
The result is successful if the OpcLabs.BaseLib.OperationModel.OperationResult.Exception is a null reference. Otherwise, this property contains information about the reason of the failure.
In OPC UA Data Access, reading data from attributes of OPC nodes is one of the most common tasks. The OPC server generally provides data for any OPC attribute in form of a Value, Timestamps and Status Code combination (see chapter “OPC Attribute Data”).
If you want to read the current attribute data from an attribute of an OPC node, call the Read method. You pass in individual arguments for the endpoint descriptor and the node ID. You will receive back a UAAttributeData object holding the current value, timestamps, and status code for the specified OPC node and attribute. The Read method returns the current attribute data, regardless of the actual status code. You may receive an Uncertain or even Bad status (and no usable data value), and your code needs to deal with such situations accordingly.
If the attribute ID is not specified in the method call, the method will read from the Value attribute. There are also other overloads of the Read method that allow you to pass in the arguments in a different way, and with more information in them. For example, you can pass in a UANodeArguments object, and an attribute ID.
For reading attribute data of multiple attributes (of the same node or of different nodes) simultaneously in an efficient manner, call the ReadMultiple method (instead of multiple Read calls in a loop). You pass in an array of UAReadArguments objects, and you will receive back an array of UAAttributeDataResult objects.
OPC “Classic” has separate functions for reading so-called OPC properties. OPC properties contain additional information related to a node. In OPC Unified Architecture, properties are accessed in the same way as other information. That is, if you have a node ID (obtained e.g. by browsing) of a property, you can use one of the ReadXXXX methods described in this chapter to get a value (or some other attribute) of that property.
The details of how the read will be performed are specified using the read parameters object (UAReadParameters class). In the most generic case, all arguments for the read method are contained in the UAReadArguments object, and the read parameters are in its ReadParameters property. There are, however, many extension methods for reading, and some of the accept the UAReadParameters object as an argument directly.
It is possible to specify the maximum age of the value to be read. In order to do that, set the MaximumAge property of the read parameters to the appropriate number of milliseconds. The MaximumAge property corresponds to the maxAge parameter in the OPC UA Read Service request, and you can read more details about it here: Table 53 - Read Service Parameters.
By default, the read parameters are set to UAReadParameters.FromCache, which denotes reading from the (OPC server) cache (and using the default encoding), and corresponds to MaximumAge equal to the CacheMaximumAge constant (Int32.MaxValue). Setting the read parameters to UAReadParameters.FromDevice will cause the server to read from the device (data source), and use the default encoding. Reading from the device is achieved by setting the MaximumAge to the DeviceMaximumAge constant (which is, in fact, zero).
For choosing a specific maximum age (in milliseconds), set the MaximumAge property to a value between zero and Int32.MaxValue. In .NET languages, there is an implicit conversion operator from Double to UAReadParameters, which means that you can simply use the number of milliseconds that represents the maximum age in place of any argument that expects the read parameters (the UAReadParameters object). There is also a FromDouble Method which has the same functionality as the conversion operator.
The default setting of read parameters, which specifies reading from the cache, may sometimes "bite" you, if you expect to receive an up-to-date value from the data source. Furthermore, you may even receive a "bad quality" indication with some first reads, because the (OPC server) cache is not yet filled with valid data. Make sure to specify the proper maximum age for the read operation, if the default behavior is not what you want. The default cannot be set to reading from the device (data source), because reading from the device (or using very short maximum age) is an ineffective practice that is discouraged, and should only be used when absolutely necessary. And, choosing a concrete number of milliseconds as a default for the maximum age would be sub-optimal, because the application requirements differ greatly, and there is no maximum age that "fits them all".
Be aware that it is physically impossible for any system to always obtain fully up-to-date values all the time.
The read parameters also allow you to select the encoding that will be used to transfer the value (e.g. binary, or XML). This is done using the EncodingName Property of the read parameters, which is a UAQualifiedName. The default is a null qualified name, which means that the encoding will be selected automatically. If you want a specific encoding be used (and the OPC server supports it), you can set this property to e.g. UABrowseNames.DefaultBinary or UABrowseNames.DefaultXml. In .NET languages, there is an implicit conversion operator from UAQualifiedName to UAReadParameters, which means that you can simply use the browse name of the encoding in place of any argument that expects the read parameters (the UAReadParameters object). There is also a FromUAQualifiedName Method which has the same functionality as the conversion operator.
If you access some node or nodes repeatedly, it might be possible to improve the performance of it by (pre-)registering the node or nodes with the server. The performance improvement will only occur if the target OPC UA server supports the necessary node registration services. For more information, see OPC UA Node Registration Service.
// This example shows how to read data (value, timestamps, and status code) of 3 attributes at once. In this example, // we are reading a Value attribute of 3 different nodes, but the method can also be used to read multiple attributes // of the same node. // // Find all latest examples here: https://opclabs.doc-that.com/files/onlinedocs/OPCLabs-OpcStudio/Latest/examples.html . // OPC client and subscriber examples in C# on GitHub: https://github.com/OPCLabs/Examples-QuickOPC-CSharp . // Missing some example? Ask us for it on our Online Forums, https://www.opclabs.com/forum/index ! You do not have to own // a commercial license in order to use Online Forums, and we reply to every post. using System; using OpcLabs.EasyOpc.UA; using OpcLabs.EasyOpc.UA.OperationModel; namespace UADocExamples._EasyUAClient { partial class ReadMultiple { public static void Main1() { UAEndpointDescriptor endpointDescriptor = "opc.tcp://opcua.demo-this.com:51210/UA/SampleServer"; // or "http://opcua.demo-this.com:51211/UA/SampleServer" (currently not supported) // or "https://opcua.demo-this.com:51212/UA/SampleServer/" // Instantiate the client object. var client = new EasyUAClient(); // Obtain attribute data. By default, the Value attributes of the nodes will be read. UAAttributeDataResult[] attributeDataResultArray = client.ReadMultiple(new[] { new UAReadArguments(endpointDescriptor, "nsu=http://test.org/UA/Data/ ;i=10845"), new UAReadArguments(endpointDescriptor, "nsu=http://test.org/UA/Data/ ;i=10853"), new UAReadArguments(endpointDescriptor, "nsu=http://test.org/UA/Data/ ;i=10855") }); // Display results. foreach (UAAttributeDataResult attributeDataResult in attributeDataResultArray) { if (attributeDataResult.Succeeded) Console.WriteLine($"AttributeData: {attributeDataResult.AttributeData}"); else Console.WriteLine($"*** Failure: {attributeDataResult.ErrorMessageBrief}"); } } // Example output: // //AttributeData: 51 {Int16} @11/6/2011 1:49:19 PM @11/6/2011 1:49:19 PM; Good //AttributeData: -1993984 {Single} @11/6/2011 1:49:19 PM @11/6/2011 1:49:19 PM; Good //AttributeData: Yellow% Dragon Cat) White Blue Dog# Green Banana- {String} @11/6/2011 1:49:19 PM @11/6/2011 1:49:19 PM; Good } }
# This example shows how to read data (value, timestamps, and status code) of 3 attributes at once. In this example, # we are reading a Value attribute of 3 different nodes, but the method can also be used to read multiple attributes # of the same node. # # Find all latest examples here: https://opclabs.doc-that.com/files/onlinedocs/OPCLabs-OpcStudio/Latest/examples.html . # OPC client and subscriber examples in PowerShell on GitHub: https://github.com/OPCLabs/Examples-QuickOPC-PowerShell . # Missing some example? Ask us for it on our Online Forums, https://www.opclabs.com/forum/index ! You do not have to own # a commercial license in order to use Online Forums, and we reply to every post. #requires -Version 5.1 using namespace OpcLabs.EasyOpc.UA using namespace OpcLabs.EasyOpc.UA.OperationModel # The path below assumes that the current directory is [ProductDir]/Examples-NET/PowerShell/Windows . Add-Type -Path "../../../Components/Opclabs.QuickOpc/net472/OpcLabs.EasyOpcUA.dll" Add-Type -Path "../../../Components/Opclabs.QuickOpc/net472/OpcLabs.EasyOpcUAComponents.dll" [UAEndpointDescriptor]$endpointDescriptor = "opc.tcp://opcua.demo-this.com:51210/UA/SampleServer" # or "http://opcua.demo-this.com:51211/UA/SampleServer" (currently not supported) # or "https://opcua.demo-this.com:51212/UA/SampleServer/" # Instantiate the client object. $client = New-Object EasyUAClient # Obtain attribute data. By default, the Value attributes of the nodes will be read. $attributeDataResultArray = $client.ReadMultiple(@( (New-Object UAReadArguments($endpointDescriptor, "nsu=http://test.org/UA/Data/ ;i=10845")), (New-Object UAReadArguments($endpointDescriptor, "nsu=http://test.org/UA/Data/ ;i=10853")), (New-Object UAReadArguments($endpointDescriptor, "nsu=http://test.org/UA/Data/ ;i=10855")) )) foreach ($attributeDataResult in $attributeDataResultArray) { if ($attributeDataResult.Succeeded) { Write-Host "AttributeData: $($attributeDataResult.AttributeData)" } else { Write-Host "*** Failure: $($attributeDataResult.ErrorMessageBrief)" } } # Example output: # #AttributeData: 51 {Int16} @11/6/2011 1:49:19 PM @11/6/2011 1:49:19 PM; Good #AttributeData: -1993984 {Single} @11/6/2011 1:49:19 PM @11/6/2011 1:49:19 PM; Good #AttributeData: Yellow% Dragon Cat) White Blue Dog# Green Banana- {String} @11/6/2011 1:49:19 PM @11/6/2011 1:49:19 PM; Good
' This example shows how to read data (value, timestamps, and status code) of 3 attributes at once. In this example, ' we are reading a Value attribute of 3 different nodes, but the method can also be used to read multiple attributes ' of the same node. ' ' Find all latest examples here: https://opclabs.doc-that.com/files/onlinedocs/OPCLabs-OpcStudio/Latest/examples.html . ' OPC client and subscriber examples in VB.NET on GitHub: https://github.com/OPCLabs/Examples-QuickOPC-VBNET . ' Missing some example? Ask us for it on our Online Forums, https://www.opclabs.com/forum/index ! You do not have to own ' a commercial license in order to use Online Forums, and we reply to every post. Imports OpcLabs.EasyOpc.UA Imports OpcLabs.EasyOpc.UA.OperationModel Namespace _EasyUAClient Partial Friend Class ReadMultiple Public Shared Sub Main1() ' Define which server we will work with. Dim endpointDescriptor As UAEndpointDescriptor = "opc.tcp://opcua.demo-this.com:51210/UA/SampleServer" ' or "http://opcua.demo-this.com:51211/UA/SampleServer" (currently not supported) ' or "https://opcua.demo-this.com:51212/UA/SampleServer/" ' Instantiate the client object Dim client = New EasyUAClient() ' Obtain attribute data. By default, the Value attributes of the nodes will be read. Dim attributeDataResultArray() As UAAttributeDataResult = client.ReadMultiple(New UAReadArguments() _ { New UAReadArguments(endpointDescriptor, "nsu=http://test.org/UA/Data/ ;i=10845"), New UAReadArguments(endpointDescriptor, "nsu=http://test.org/UA/Data/ ;i=10853"), New UAReadArguments(endpointDescriptor, "nsu=http://test.org/UA/Data/ ;i=10855") }) ' Display results For Each attributeDataResult As UAAttributeDataResult In attributeDataResultArray If attributeDataResult.Succeeded Then Console.WriteLine("AttributeData: {0}", attributeDataResult.AttributeData) Else Console.WriteLine("*** Failure: {0}", attributeDataResult.ErrorMessageBrief) End If Next attributeDataResult ' Example output: ' 'AttributeData: 51 {System.Int16} @11/6/2011 1:49:19 PM @11/6/2011 1:49:19 PM; Good 'AttributeData: -1993984 {System.Single} @11/6/2011 1:49:19 PM @11/6/2011 1:49:19 PM; Good 'AttributeData: Yellow% Dragon Cat) White Blue Dog# Green Banana- {System.String} @11/6/2011 1:49:19 PM @11/6/2011 1:49:19 PM; Good End Sub End Class End Namespace
# This example shows how to read data (value, timestamps, and status code) of 3 attributes at once. In this example, # we are reading a Value attribute of 3 different nodes, but the method can also be used to read multiple attributes # of the same node. # # Find all latest examples here: https://opclabs.doc-that.com/files/onlinedocs/OPCLabs-OpcStudio/Latest/examples.html . # OPC client and subscriber examples in Python on GitHub: https://github.com/OPCLabs/Examples-QuickOPC-Python . # Missing some example? Ask us for it on our Online Forums, https://www.opclabs.com/forum/index ! You do not have to own # a commercial license in order to use Online Forums, and we reply to every post. # The QuickOPC package is needed. Install it using "pip install opclabs_quickopc". import opclabs_quickopc import time # Import .NET namespaces. from OpcLabs.EasyOpc.UA import * from OpcLabs.EasyOpc.UA.OperationModel import * endpointDescriptor = UAEndpointDescriptor('opc.tcp://opcua.demo-this.com:51210/UA/SampleServer') # or 'http://opcua.demo-this.com:51211/UA/SampleServer' (currently not supported) # or 'https://opcua.demo-this.com:51212/UA/SampleServer/' # Instantiate the client object. client = EasyUAClient() # Obtain attribute data. By default, the Value attributes of the nodes will be read. attributeDataResultArray = client.ReadMultiple([ UAReadArguments(endpointDescriptor, UANodeDescriptor('nsu=http://test.org/UA/Data/ ;i=10845')), UAReadArguments(endpointDescriptor, UANodeDescriptor('nsu=http://test.org/UA/Data/ ;i=10853')), UAReadArguments(endpointDescriptor, UANodeDescriptor('nsu=http://test.org/UA/Data/ ;i=10855')), ]) # Display results. for attributeDataResult in attributeDataResultArray: if attributeDataResult.Succeeded: print('AttributeData: ', attributeDataResult.AttributeData, sep='') else: print('*** Failure: ', attributeDataResult.ErrorMessageBrief, sep='') print() print('Finished.')
System.Object
OpcLabs.BaseLib.Object2
OpcLabs.BaseLib.Info
OpcLabs.BaseLib.OperationModel.OperationResult
OpcLabs.EasyOpc.UA.OperationModel.UAAttributeDataResult
OpcLabs.EasyOpc.UA.Generic.UAAttributeDataResult<T>