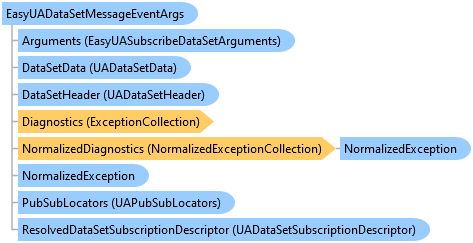
'Declaration
<CLSCompliantAttribute(True)> <ComDefaultInterfaceAttribute(OpcLabs.EasyOpc.UA.PubSub.OperationModel.ComTypes._EasyUADataSetMessageEventArgs)> <ComVisibleAttribute(True)> <GuidAttribute("AB4ADB6F-E7CC-4FEB-91FF-A2A84E738B13")> <TypeConverterAttribute(System.ComponentModel.ExpandableObjectConverter)> <SerializableAttribute()> Public Class EasyUADataSetMessageEventArgs Inherits EasyUAPubSubMessageEventArgs Implements OpcLabs.BaseLib.OperationModel.ComTypes._OperationEventArgs, OpcLabs.EasyOpc.UA.PubSub.OperationModel.ComTypes._EasyUADataSetMessageEventArgs, OpcLabs.EasyOpc.UA.PubSub.OperationModel.ComTypes._EasyUAPubSubMessageEventArgs, System.ICloneable, System.Runtime.Serialization.ISerializable, System.Xml.Serialization.IXmlSerializable
'Usage
Dim instance As EasyUADataSetMessageEventArgs
[CLSCompliant(true)] [ComDefaultInterface(OpcLabs.EasyOpc.UA.PubSub.OperationModel.ComTypes._EasyUADataSetMessageEventArgs)] [ComVisible(true)] [Guid("AB4ADB6F-E7CC-4FEB-91FF-A2A84E738B13")] [TypeConverter(System.ComponentModel.ExpandableObjectConverter)] [Serializable()] public class EasyUADataSetMessageEventArgs : EasyUAPubSubMessageEventArgs, OpcLabs.BaseLib.OperationModel.ComTypes._OperationEventArgs, OpcLabs.EasyOpc.UA.PubSub.OperationModel.ComTypes._EasyUADataSetMessageEventArgs, OpcLabs.EasyOpc.UA.PubSub.OperationModel.ComTypes._EasyUAPubSubMessageEventArgs, System.ICloneable, System.Runtime.Serialization.ISerializable, System.Xml.Serialization.IXmlSerializable
[CLSCompliant(true)] [ComDefaultInterface(OpcLabs.EasyOpc.UA.PubSub.OperationModel.ComTypes._EasyUADataSetMessageEventArgs)] [ComVisible(true)] [Guid("AB4ADB6F-E7CC-4FEB-91FF-A2A84E738B13")] [TypeConverter(System.ComponentModel.ExpandableObjectConverter)] [Serializable()] public ref class EasyUADataSetMessageEventArgs : public EasyUAPubSubMessageEventArgs, OpcLabs.BaseLib.OperationModel.ComTypes._OperationEventArgs, OpcLabs.EasyOpc.UA.PubSub.OperationModel.ComTypes._EasyUADataSetMessageEventArgs, OpcLabs.EasyOpc.UA.PubSub.OperationModel.ComTypes._EasyUAPubSubMessageEventArgs, System.ICloneable, System.Runtime.Serialization.ISerializable, System.Xml.Serialization.IXmlSerializable
If you are on Windows, you can also use the OPC UA PubSub Demo Application to experiment with various settings. This allows you to try out and determine the correct parameters before you put them into the code.
A subscription is initiated by calling the SubscribeDataSet Method on the EasyUASubscriber Class. In its basic form, the method takes a single argument which is an instance of the EasyUASubscribeDataSetArguments Class, and this argument contains all the information necessary to perform the subscription. The object and all the sub-objects it contains are rather complex.
The picture below is meant to give you at least a general overview about what this object contains. There are more "smaller" properties to the individual objects on the picture, but we do not care about them for now.
The main parts of the EasyUASubscribeDataSetArguments is the DataSetSubscriptionDescriptor: it describes the dataset(s) you want to subscribe to.
The ResolverDescriptor in the DataSetSubscriptionDescriptor is for OPC UA PubSub logical resolution, if used.
Inside the dataset subscription descriptor, you always need to specify the PubSub connection, using the ConnectionDescriptor Property. You will typically also need to fill in data in the Filter Property (see Message Filtering (OPC UA PubSub)), and sometimes provide the DataSetMetaData Property (see Dataset Metadata (OPC UA PubSub)). The CommunicationParameters Property is used to specify details about the communication, message receive timeout, and parameters specific to message or transport protocol mapping.
There are many (extension method) overloads of the SubscribeDataSet Method, with various combinations of arguments. These overloads allow you to bypass the construction of the whole EasyUASubscribeDataSetArguments hierarchy, and pass in simply the parts you need.
The example below subscribes to all dataset messages on an OPC-UA PubSub connection with UDP UADP mapping. The connection is specified by its physical parameters, using the scheme name "opc.udp" and the IP address of the multicast group to listen on.
The example specifies just the PubSub connection, and receives all PubSub messages it encounters. In .NET, it illustrates the use of a SubscribeDataSet Method overload that takes just the PubSub connection descriptor as an input.
The information you pass in to the SubscribeDataSet Method may either be used "as is", or it may be subject to OPC UA PubSub logical resolution first. As explained in OPC UA PubSub Descriptors, you can pass in the physical identifiers, the logical identifiers, or both. The OPC UA PubSub logical resolution mechanism will be invoked if the dataset subscription descriptor requires resolution (this term is explained in OPC UA PubSub Descriptors).
We can reformulate it so: If you have specified physical identifiers everywhere, they will be directly used, and no logical resolution will take place - even if there are logical identifies filled in as well. If, however, you have a PubSub object somewhere in the descriptors that is only specified by its logical identifier but no physical identifier, the logical resolution mechanism will be used.
There are two ways your program can be informed about dataset messages that you have subscribed to:
It is also possible to specify a non-null callback parameter, and hook an event handler as well. In this case, the callback method will be invoked in addition to the event handlers.
The event arguments of the DataSetMessage Event are of type EasyUADataSetMessageEventArgs. Because it is indirectly derived from the OperationEventArgs, it contains the Exception Property which is null for success notifications, and non-null in case of failure. You can use the Succeeded Property to make the corresponding test. The actual data of the dataset, if available, is in the DataSetData Property.
The EasyUADataSetMessageEventArgs also contain, in their Arguments Property, a copy of the arguments (EasyUASubscribeDataSetArguments Class) you have used when making the dataset subscription that caused this event or callback. You can use this property to identify the dataset subscription, in case you are using event handlers and have made more subscriptions on the same object, or if you have used the same callback methods with multiple subscriptions. Specifically, the State Property in the EasyUASubscribeDataSetArguments can be used for any information you need to pass from the code that makes the subscription to the code that handles the dataset messages.
The EasyUASubscriber invokes the DataSetMessage event or callback when a new dataset message (you have subscribed to) arrives, but also in some other cases. Below is a list of possible property value combinations and their meaning.
The main causes for failures are
If the OPC UA PubSub logical resolution took place, the ResolvedDataSetSubscriptionDescriptor Property is filled in with a dataset subscription descriptor that is derived from the one you have specified when calling the SubscribeDataSet Method, but with the logical identifiers resolved to physical ones.
The dataset message event notification has a PubSubLocators Property. This property is always non-null (even in case of errors), and contains the information that allows to relate the event to its "source" in the OPC UA PubSub model. The object (UAPubSubLocators Class) has properties for a publisher Id, writer group Id, writer group name, dataset writer Id, and dataset writer name. The component will fill in as many of these properties as possible, given the circumstances. Knowing such relation comes handy when the dataset subscription filter is not for a single dataset, but for multiple datasets. Note that all information contained in the PubSubLocators Property is also available inside the dataset data. The difference is that the PubSubLocators object is present even in cases when there is dataset data - such as in case of errors. The PubSubLocators thus allow to determine the "source" of the error programmatically.
A typical task when processing the dataset message is to extract one or more fields from the dataset and process them further. The field is identified by its name (or an index in its string form, such "#0", if field names are not available) in the FieldDataDictionary. The example below shows how it can be done.
If metadata is available, you can alternatively use the FieldDataDictionaryById Property, which also contains the data for each field of the dataset message, but keyed by a dataset field Id (a GUID).
Depending how OPC UA PubSub is configured, the publisher might be always sending all fields of the dataset with every dataset message, or it may use a combination of key frames and delta frames. While the key frame dataset message includes values for all fields of the dataset, the delta frame only contains values of the fields that have changed since the previous dataset message.
Key frames and delta frames are a concept that exists purely to spare the network bandwidth. When you subscribe with OPC Data Client, the delta frames are interpreted internally, and a full dataset is always shipped to you in the dataset message event or callback. This way, you do not have to put in any additional code to handle the distinction between key frames and delta frames.
It is not necessary to unsubscribe and subscribe again, if you want to change some parameters of existing subscription, such as its filter (Message Filtering (OPC UA PubSub)) or the associated metadata (Dataset Metadata (OPC UA PubSub)). You change the parameters by calling the ChangeDataSetSubscription Method, passing it the dataset subscription handle, and the new parameters in form of an instance of the EasyUADataSetSubscriptionChangeArguments Class.
There is also an extension method (an overload - with the same name) that allows you to easily pass in just the dataset subscription handle, and a new filter.
If you no longer want to receive dataset messages, you need to unsubscribe. This is achieved by calling the UnsubscribeDataSet Method, passing it the dataset subscription handle obtained when calling the SubscribeDataSet Method.
Example:
You can also unsubscribe from all dataset messages you have previously subscribed to (on the same instance of the EasyUASubscriber Class) by calling the UnsubscribeAllDataSets Method.
OPC Data Client merges together requests to the same PubSub objects. If, for example, you make multiple dataset subscriptions and they all use the same PubSub connection, OPC Data Client will only make the connection once.
This merging is performed among all subscriptions on all EasyUASubscriber objects that have their Isolated Property set to 'false' (the default). If you set the Isolated Property on some EasyUASubscriber object to 'true', the merging is then performed on the subscriptions made on that object only.
Newer versions of OPC UA specification allow the publishers to inform interested parties about their status, either cyclically, or when the status changes. OPC Studio recognizes and processes the publisher status messages in UADP and JSON message mappings. Any non-operational publisher status is reported as an error through the dataset subscription event handler or callback.
This functionality is commonly used with OPC UA PubSub Topic Tree, in which case the topic tree rules prescribe the name of the topic where the status messages for a particular publisher are located.
// This example shows how to subscribe to all dataset messages on an OPC-UA PubSub connection with UDP UADP mapping. // // In order to produce network messages for this example, run the UADemoPublisher tool. For documentation, see // https://kb.opclabs.com/UADemoPublisher_Basics . In some cases, you may have to specify the interface name to be used. // // Find all latest examples here: https://opclabs.doc-that.com/files/onlinedocs/OPCLabs-OpcStudio/Latest/examples.html . // OPC client and subscriber examples in C# on GitHub: https://github.com/OPCLabs/Examples-QuickOPC-CSharp . // Missing some example? Ask us for it on our Online Forums, https://www.opclabs.com/forum/index ! You do not have to own // a commercial license in order to use Online Forums, and we reply to every post. using System; using System.Collections.Generic; using System.Threading; using OpcLabs.EasyOpc.UA.PubSub; using OpcLabs.EasyOpc.UA.PubSub.OperationModel; namespace UASubscriberDocExamples.PubSub._EasyUASubscriber { partial class SubscribeDataSet { public static void Main1() { // Define the PubSub connection we will work with. Uses implicit conversion from a string. UAPubSubConnectionDescriptor pubSubConnectionDescriptor = "opc.udp://239.0.0.1"; // In some cases you may have to set the interface (network adapter) name that needs to be used, similarly to // the statement below. Your actual interface name may differ, of course. //pubSubConnectionDescriptor.ResourceAddress.InterfaceName = "Ethernet"; // Instantiate the subscriber object and hook events. var subscriber = new EasyUASubscriber(); subscriber.DataSetMessage += subscriber_DataSetMessage; Console.WriteLine("Subscribing..."); subscriber.SubscribeDataSet(pubSubConnectionDescriptor); Console.WriteLine("Processing dataset message events for 20 seconds..."); Thread.Sleep(20 * 1000); Console.WriteLine("Unsubscribing..."); subscriber.UnsubscribeAllDataSets(); Console.WriteLine("Waiting for 1 second..."); // Unsubscribe operation is asynchronous, messages may still come for a short while. Thread.Sleep(1 * 1000); Console.WriteLine("Finished."); } static void subscriber_DataSetMessage(object sender, EasyUADataSetMessageEventArgs e) { // Display the dataset. if (e.Succeeded) { // An event with null DataSetData just indicates a successful connection. if (!(e.DataSetData is null)) { Console.WriteLine(); Console.WriteLine($"Dataset data: {e.DataSetData}"); foreach (KeyValuePair<string, UADataSetFieldData> pair in e.DataSetData.FieldDataDictionary) Console.WriteLine(pair); } } else { Console.WriteLine(); Console.WriteLine($"*** Failure: {e.ErrorMessageBrief}"); } } // Example output (truncated): // //Subscribing... //Processing dataset message events for 20 seconds... // //Dataset data: Good; Data; publisher="32", writer=1, class=eae79794-1af7-4f96-8401-4096cd1d8908, fields: 4 //[#0, True {System.Boolean} @0001-01-01T00:00:00.000 @@0001-01-01T00:00:00.000; Good] //[#1, 7945 {System.Int32} @0001-01-01T00:00:00.000 @@0001-01-01T00:00:00.000; Good] //[#2, 5246 {System.Int32} @0001-01-01T00:00:00.000 @@0001-01-01T00:00:00.000; Good] //[#3, 9/30/2019 11:19:14 AM {System.DateTime} @0001-01-01T00:00:00.000 @@0001-01-01T00:00:00.000; Good] // //Dataset data: Good; Data; publisher="32", writer=3, class=96976b7b-0db7-46c3-a715-0979884b55ae, fields: 100 //[#0, 45 {System.Int64} @0001-01-01T00:00:00.000 @@0001-01-01T00:00:00.000; Good] //[#1, 145 {System.Int64} @0001-01-01T00:00:00.000 @@0001-01-01T00:00:00.000; Good] //[#2, 245 {System.Int64} @0001-01-01T00:00:00.000 @@0001-01-01T00:00:00.000; Good] //[#3, 345 {System.Int64} @0001-01-01T00:00:00.000 @@0001-01-01T00:00:00.000; Good] //[#4, 445 {System.Int64} @0001-01-01T00:00:00.000 @@0001-01-01T00:00:00.000; Good] //[#5, 545 {System.Int64} @0001-01-01T00:00:00.000 @@0001-01-01T00:00:00.000; Good] //[#6, 645 {System.Int64} @0001-01-01T00:00:00.000 @@0001-01-01T00:00:00.000; Good] //[#7, 745 {System.Int64} @0001-01-01T00:00:00.000 @@0001-01-01T00:00:00.000; Good] //[#8, 845 {System.Int64} @0001-01-01T00:00:00.000 @@0001-01-01T00:00:00.000; Good] //[#9, 945 {System.Int64} @0001-01-01T00:00:00.000 @@0001-01-01T00:00:00.000; Good] //[#10, 1045 {System.Int64} @0001-01-01T00:00:00.000 @@0001-01-01T00:00:00.000; Good] //... } }
# This example shows how to subscribe to all dataset messages on an OPC-UA PubSub connection with UDP UADP mapping. # # In order to produce network messages for this example, run the UADemoPublisher tool. For documentation, see # http:#kb.opclabs.com/UADemoPublisher_Basics . In some cases, you may have to specify the interface name to be used. # # Find all latest examples here: https://opclabs.doc-that.com/files/onlinedocs/OPCLabs-OpcStudio/Latest/examples.html . # OPC client and subscriber examples in PowerShell on GitHub: https://github.com/OPCLabs/Examples-QuickOPC-PowerShell . # Missing some example? Ask us for it on our Online Forums, https://www.opclabs.com/forum/index ! You do not have to own # a commercial license in order to use Online Forums, and we reply to every post. #requires -Version 5.1 using namespace OpcLabs.BaseLib.Networking using namespace OpcLabs.EasyOpc.UA using namespace OpcLabs.EasyOpc.UA.PubSub using namespace OpcLabs.EasyOpc.UA.PubSub.OperationModel # The path below assumes that the current directory is [ProductDir]/Examples-NET/PowerShell/Windows . Add-Type -Path "../../../Components/Opclabs.QuickOpc/net472/OpcLabs.EasyOpcUA.dll" Add-Type -Path "../../../Components/Opclabs.QuickOpc/net472/OpcLabs.EasyOpcUAComponents.dll" # Define the PubSub connection we will work with. Uses implicit conversion from a string. [UAPubSubConnectionDescriptor]$pubSubConnectionDescriptor = [ResourceAddress]"opc.udp://239.0.0.1" # In some cases you may have to set the interface (network adapter) name that needs to be used, similarly to # the statement below. Your actual interface name may differ, of course. #$pubSubConnectionDescriptor.ResourceAddress.InterfaceName = "Ethernet" # Instantiate the subscriber object. $subscriber = New-Object EasyUASubscriber # Event notification handler Register-ObjectEvent -InputObject $subscriber -EventName DataSetMessage -Action { # Display the dataset. if ($EventArgs.Succeeded) { # An event with null DataSetData just indicates a successful connection. if ($EventArgs.DataSetData -ne $null) { Write-Host Write-Host "Dataset data: $($EventArgs.DataSetData)" foreach ($pair in $EventArgs.DataSetData.FieldDataDictionary.GetEnumerator()) { Write-Host $pair } } } else { Write-Host Write-Host "*** Failure: $($EventArgs.ErrorMessageBrief)" } } Write-Host "Subscribing..." [IEasyUASubscriberExtension]::SubscribeDataSet($subscriber, $pubSubConnectionDescriptor) Write-Host "Processing dataset message events for 20 seconds..." $stopwatch = [System.Diagnostics.Stopwatch]::StartNew() while ($stopwatch.Elapsed.TotalSeconds -lt 20) { Start-Sleep -Seconds 1 } Write-Host "Unsubscribing..." $subscriber.UnsubscribeAllDataSets() Write-Host "Waiting for 1 second..." # Unsubscribe operation is asynchronous, messages may still come for a short while. Start-Sleep -Seconds 1 Write-Host "Finished." # Example output (truncated): # #Subscribing... #Processing dataset message events for 20 seconds... # #Dataset data: Good; Data; publisher="32", writer=1, class=eae79794-1af7-4f96-8401-4096cd1d8908, fields: 4 #[#0, True {System.Boolean} @0001-01-01T00:00:00.000 @@0001-01-01T00:00:00.000; Good] #[#1, 7945 {System.Int32} @0001-01-01T00:00:00.000 @@0001-01-01T00:00:00.000; Good] #[#2, 5246 {System.Int32} @0001-01-01T00:00:00.000 @@0001-01-01T00:00:00.000; Good] #[#3, 9/30/2019 11:19:14 AM {System.DateTime} @0001-01-01T00:00:00.000 @@0001-01-01T00:00:00.000; Good] # #Dataset data: Good; Data; publisher="32", writer=3, class=96976b7b-0db7-46c3-a715-0979884b55ae, fields: 100 #[#0, 45 {System.Int64} @0001-01-01T00:00:00.000 @@0001-01-01T00:00:00.000; Good] #[#1, 145 {System.Int64} @0001-01-01T00:00:00.000 @@0001-01-01T00:00:00.000; Good] #[#2, 245 {System.Int64} @0001-01-01T00:00:00.000 @@0001-01-01T00:00:00.000; Good] #[#3, 345 {System.Int64} @0001-01-01T00:00:00.000 @@0001-01-01T00:00:00.000; Good] #[#4, 445 {System.Int64} @0001-01-01T00:00:00.000 @@0001-01-01T00:00:00.000; Good] #[#5, 545 {System.Int64} @0001-01-01T00:00:00.000 @@0001-01-01T00:00:00.000; Good] #[#6, 645 {System.Int64} @0001-01-01T00:00:00.000 @@0001-01-01T00:00:00.000; Good] #[#7, 745 {System.Int64} @0001-01-01T00:00:00.000 @@0001-01-01T00:00:00.000; Good] #[#8, 845 {System.Int64} @0001-01-01T00:00:00.000 @@0001-01-01T00:00:00.000; Good] #[#9, 945 {System.Int64} @0001-01-01T00:00:00.000 @@0001-01-01T00:00:00.000; Good] #[#10, 1045 {System.Int64} @0001-01-01T00:00:00.000 @@0001-01-01T00:00:00.000; Good] #...
' This example shows how to subscribe to all dataset messages on an OPC-UA PubSub connection with UDP UADP mapping. ' ' In order to produce network messages for this example, run the UADemoPublisher tool. For documentation, see ' https://kb.opclabs.com/UADemoPublisher_Basics . In some cases, you may have to specify the interface name to be used. ' ' Find all latest examples here: https://opclabs.doc-that.com/files/onlinedocs/OPCLabs-OpcStudio/Latest/examples.html . ' OPC client and subscriber examples in VB.NET on GitHub: https://github.com/OPCLabs/Examples-QuickOPC-VBNET . ' Missing some example? Ask us for it on our Online Forums, https://www.opclabs.com/forum/index ! You do not have to own ' a commercial license in order to use Online Forums, and we reply to every post. Imports OpcLabs.EasyOpc.UA.PubSub Imports OpcLabs.EasyOpc.UA.PubSub.OperationModel Namespace PubSub._EasyUASubscriber Friend Class SubscribeDataSet Public Shared Sub Main1() ' Define the PubSub connection we will work with. Uses implicit conversion from a string. Dim pubSubConnectionDescriptor As UAPubSubConnectionDescriptor = "opc.udp://239.0.0.1" ' In some cases you may have to set the interface (network adapter) name that needs to be used, similarly to ' the statement below. Your actual interface name may differ, of course. ' pubSubConnectionDescriptor.ResourceAddress.InterfaceName = "Ethernet" ' Instantiate the subscriber object and hook events. Dim subscriber = New EasyUASubscriber() AddHandler subscriber.DataSetMessage, AddressOf subscriber_DataSetMessage Console.WriteLine("Subscribing...") subscriber.SubscribeDataSet(pubSubConnectionDescriptor) Console.WriteLine("Processing dataset message events for 20 seconds...") Threading.Thread.Sleep(20 * 1000) Console.WriteLine("Unsubscribing...") subscriber.UnsubscribeAllDataSets() Console.WriteLine("Waiting for 1 second...") ' Unsubscribe operation is asynchronous, messages may still come for a short while. Threading.Thread.Sleep(1 * 1000) Console.WriteLine("Finished...") End Sub Private Shared Sub subscriber_DataSetMessage(ByVal sender As Object, ByVal e As EasyUADataSetMessageEventArgs) ' Display the dataset. If e.Succeeded Then ' An event with null DataSetData just indicates a successful connection. If e.DataSetData IsNot Nothing Then Console.WriteLine() Console.WriteLine($"Dataset data: {e.DataSetData}") For Each pair As KeyValuePair(Of String, UADataSetFieldData) In e.DataSetData.FieldDataDictionary Console.WriteLine(pair) Next End If Else Console.WriteLine() Console.WriteLine($"*** Failure: {e.ErrorMessageBrief}") End If End Sub End Class ' Example output ' 'Subscribing... 'Processing dataset message events for 20 seconds... ' ''Dataset data: Good; Data; publisher="32", writer=1, class=eae79794-1af7-4f96-8401-4096cd1d8908, fields: 4 '[#0, True {System.Boolean} @0001-01-01T00:00:00.000 @@0001-01-01T00:00:00.000; Good] '[#1, 7945 {System.Int32} @0001-01-01T00:00:00.000 @@0001-01-01T00:00:00.000; Good] '[#2, 5246 {System.Int32} @0001-01-01T00:00:00.000 @@0001-01-01T00:00:00.000; Good] '[#3, 9/30/2019 11:19:14 AM {System.DateTime} @0001-01-01T00:00:00.000 @@0001-01-01T00:00:00.000; Good] ' 'Dataset data: Good; Data; publisher="32", writer=3, class=96976b7b-0db7-46c3-a715-0979884b55ae, fields: 100 '[#0, 45 {System.Int64} @0001-01-01T00:00:00.000 @@0001-01-01T00:00:00.000; Good] '[#1, 145 {System.Int64} @0001-01-01T00:00:00.000 @@0001-01-01T00:00:00.000; Good] '[#2, 245 {System.Int64} @0001-01-01T00:00:00.000 @@0001-01-01T00:00:00.000; Good] '[#3, 345 {System.Int64} @0001-01-01T00:00:00.000 @@0001-01-01T00:00:00.000; Good] '[#4, 445 {System.Int64} @0001-01-01T00:00:00.000 @@0001-01-01T00:00:00.000; Good] '[#5, 545 {System.Int64} @0001-01-01T00:00:00.000 @@0001-01-01T00:00:00.000; Good] '[#6, 645 {System.Int64} @0001-01-01T00:00:00.000 @@0001-01-01T00:00:00.000; Good] '[#7, 745 {System.Int64} @0001-01-01T00:00:00.000 @@0001-01-01T00:00:00.000; Good] '[#8, 845 {System.Int64} @0001-01-01T00:00:00.000 @@0001-01-01T00:00:00.000; Good] '[#9, 945 {System.Int64} @0001-01-01T00:00:00.000 @@0001-01-01T00:00:00.000; Good] '[#10, 1045 {System.Int64} @0001-01-01T00:00:00.000 @@0001-01-01T00:00:00.000; Good] '... End Namespace
// This example shows how to subscribe to all dataset messages on an OPC-UA PubSub connection with UDP UADP mapping. // // In order to produce network messages for this example, run the UADemoPublisher tool. For documentation, see // https://kb.opclabs.com/UADemoPublisher_Basics . In some cases, you may have to specify the interface name to be used. // // Find all latest examples here: https://opclabs.doc-that.com/files/onlinedocs/OPCLabs-OpcStudio/Latest/examples.html . // Missing some example? Ask us for it on our Online Forums, https://www.opclabs.com/forum/index ! You do not have to own // a commercial license in order to use Online Forums, and we reply to every post. #include "stdafx.h" // Includes "QuickOpc.h", and other commonly used files #include <atlcom.h> #include "SubscribeDataSet.h" namespace PubSub { namespace _EasyUASubscriber { // CEasyUASubscriberEvents class CEasyUASubscriberEvents : public IDispEventImpl<1, CEasyUASubscriberEvents> { public: BEGIN_SINK_MAP(CEasyUASubscriberEvents) // Event handlers must have the __stdcall calling convention SINK_ENTRY(1, DISPID_EASYUASUBSCRIBEREVENTS_DATASETMESSAGE, &CEasyUASubscriberEvents::DataSetMessage) END_SINK_MAP() public: // The handler for EasyUAClient.DataChangeNotification event STDMETHOD(DataSetMessage)(VARIANT varSender, _EasyUADataSetMessageEventArgs* pEventArgs) { // Display the dataset. if (pEventArgs->Succeeded) { _UADataSetDataPtr DataSetDataPtr = pEventArgs->DataSetData; // An event with null DataSetData just indicates a successful connection. if (DataSetDataPtr != NULL) { _tprintf(_T("\n")); _tprintf(_T("Dataset data: %s\n"), (LPCTSTR)CW2CT(DataSetDataPtr->ToString)); IEnumVARIANTPtr EnumDictionaryEntry2Ptr = DataSetDataPtr->FieldDataDictionary->GetEnumerator(); _variant_t vDictionaryEntry2; while (EnumDictionaryEntry2Ptr->Next(1, &vDictionaryEntry2, NULL) == S_OK) { _DictionaryEntry2Ptr DictionaryEntry2Ptr(vDictionaryEntry2); _tprintf(_T("%s\n"), (LPCTSTR)CW2CT(DictionaryEntry2Ptr->ToString)); vDictionaryEntry2.Clear(); } } } else { _tprintf(_T("\n")); _tprintf(_T("*** Failure: %s\n"), (LPCTSTR)CW2CT(pEventArgs->ErrorMessageBrief)); } return S_OK; } }; void SubscribeDataSet::Main1() { // Initialize the COM library CoInitializeEx(NULL, COINIT_MULTITHREADED); { // Prepare arguments for a subscription to the dataset. _EasyUASubscribeDataSetArgumentsPtr SubscribeDataSetArgumentsPtr(__uuidof(EasyUASubscribeDataSetArguments)); // Define the PubSub connection we will work with. _UAPubSubConnectionDescriptorPtr PubSubConnectionDescriptorPtr = SubscribeDataSetArgumentsPtr->DataSetSubscriptionDescriptor->ConnectionDescriptor; PubSubConnectionDescriptorPtr->ResourceAddress->ResourceDescriptor->UrlString = L"opc.udp://239.0.0.1"; // In some cases you may have to set the interface (network adapter) name that needs to be used, similarly to // the statement below. Your actual interface name may differ, of course. //PubSubConnectionDescriptorPtr->ResourceAddress->InterfaceName = L"Ethernet"; // Instantiate the subscriber object. _EasyUASubscriberPtr SubscriberPtr(__uuidof(EasyUASubscriber)); // Hook events. CEasyUASubscriberEvents* pSubscriberEvents = new CEasyUASubscriberEvents(); AtlGetObjectSourceInterface(SubscriberPtr, &pSubscriberEvents->m_libid, &pSubscriberEvents->m_iid, &pSubscriberEvents->m_wMajorVerNum, &pSubscriberEvents->m_wMinorVerNum); pSubscriberEvents->m_iid = _uuidof(DEasyUASubscriberEvents); pSubscriberEvents->DispEventAdvise(SubscriberPtr, &pSubscriberEvents->m_iid); _tprintf(_T("Subscribing...\n")); _variant_t vSubscribeDataSetArguments(SubscribeDataSetArgumentsPtr.GetInterfacePtr()); SubscriberPtr->SubscribeDataSet(vSubscribeDataSetArguments); _tprintf(_T("Processing dataset message events for 20 seconds...\n")); Sleep(20 * 1000); _tprintf(_T("Unsubscribing...\n")); SubscriberPtr->UnsubscribeAllDataSets(); _tprintf(_T("Waiting for 1 second...\n")); // Unsubscribe operation is asynchronous, messages may still come for a short while. Sleep(1 * 1000); // Unhook events pSubscriberEvents->DispEventUnadvise(SubscriberPtr, &pSubscriberEvents->m_iid); _tprintf(_T("Finished.\n")); } // Release all interface pointers BEFORE calling CoUninitialize() CoUninitialize(); } // Example output: // //Subscribing... //Processing dataset message events for 20 seconds... // ////Dataset data: Good; Data; publisher="32", writer=1, class=eae79794-1af7-4f96-8401-4096cd1d8908, fields: 4 //[#0, True {System.Boolean} @0001-01-01T00:00:00.000 @@0001-01-01T00:00:00.000; Good] //[#1, 7945 {System.Int32} @0001-01-01T00:00:00.000 @@0001-01-01T00:00:00.000; Good] //[#2, 5246 {System.Int32} @0001-01-01T00:00:00.000 @@0001-01-01T00:00:00.000; Good] //[#3, 9/30/2019 11:19:14 AM {System.DateTime} @0001-01-01T00:00:00.000 @@0001-01-01T00:00:00.000; Good] // //Dataset data: Good; Data; publisher="32", writer=3, class=96976b7b-0db7-46c3-a715-0979884b55ae, fields: 100 //[#0, 45 {System.Int64} @0001-01-01T00:00:00.000 @@0001-01-01T00:00:00.000; Good] //[#1, 145 {System.Int64} @0001-01-01T00:00:00.000 @@0001-01-01T00:00:00.000; Good] //[#2, 245 {System.Int64} @0001-01-01T00:00:00.000 @@0001-01-01T00:00:00.000; Good] //[#3, 345 {System.Int64} @0001-01-01T00:00:00.000 @@0001-01-01T00:00:00.000; Good] //[#4, 445 {System.Int64} @0001-01-01T00:00:00.000 @@0001-01-01T00:00:00.000; Good] //[#5, 545 {System.Int64} @0001-01-01T00:00:00.000 @@0001-01-01T00:00:00.000; Good] //[#6, 645 {System.Int64} @0001-01-01T00:00:00.000 @@0001-01-01T00:00:00.000; Good] //[#7, 745 {System.Int64} @0001-01-01T00:00:00.000 @@0001-01-01T00:00:00.000; Good] //[#8, 845 {System.Int64} @0001-01-01T00:00:00.000 @@0001-01-01T00:00:00.000; Good] //[#9, 945 {System.Int64} @0001-01-01T00:00:00.000 @@0001-01-01T00:00:00.000; Good] //[#10, 1045 {System.Int64} @0001-01-01T00:00:00.000 @@0001-01-01T00:00:00.000; Good] //... } }
// This example shows how to subscribe to all dataset messages on an OPC-UA PubSub connection with UDP UADP mapping. // // In order to produce network messages for this example, run the UADemoPublisher tool. For documentation, see // https://kb.opclabs.com/UADemoPublisher_Basics . In some cases, you may have to specify the interface name to be used. // // Find all latest examples here: https://opclabs.doc-that.com/files/onlinedocs/OPCLabs-OpcStudio/Latest/examples.html . // OPC client and subscriber examples in Object Pascal (Delphi) on GitHub: https://github.com/OPCLabs/Examples-QuickOPC-OP . // Missing some example? Ask us for it on our Online Forums, https://www.opclabs.com/forum/index ! You do not have to own // a commercial license in order to use Online Forums, and we reply to every post. type TSubscriberEventHandlers77 = class procedure OnDataSetMessage( ASender: TObject; sender: OleVariant; const eventArgs: _EasyUADataSetMessageEventArgs); end; class procedure SubscribeDataSet.Main1; var ConnectionDescriptor: _UAPubSubConnectionDescriptor; SubscribeDataSetArguments: _EasyUASubscribeDataSetArguments; Subscriber: TEasyUASubscriber; SubscriberEventHandlers: TSubscriberEventHandlers77; begin // Define the PubSub connection we will work with. SubscribeDataSetArguments := CoEasyUASubscribeDataSetArguments.Create; ConnectionDescriptor := SubscribeDataSetArguments.DataSetSubscriptionDescriptor.ConnectionDescriptor; ConnectionDescriptor.ResourceAddress.ResourceDescriptor.UrlString := 'opc.udp://239.0.0.1'; // In some cases you may have to set the interface (network adapter) name that needs to be used, similarly to // the statement below. Your actual interface name may differ, of course. //ConnectionDescriptor.ResourceAddress.InterfaceName := 'Ethernet'; // Instantiate the subscriber object and hook events. Subscriber := TEasyUASubscriber.Create(nil); SubscriberEventHandlers := TSubscriberEventHandlers77.Create; Subscriber.OnDataSetMessage := SubscriberEventHandlers.OnDataSetMessage; WriteLn('Subscribing...'); Subscriber.SubscribeDataSet(SubscribeDataSetArguments); WriteLn('Processing dataset message for 20 seconds...'); PumpSleep(20*1000); WriteLn('Unsubscribing...'); Subscriber.UnsubscribeAllDataSets; WriteLn('Waiting for 1 second...'); // Unsubscribe operation is asynchronous, messages may still come for a short while. PumpSleep(1*1000); WriteLn('Finished.'); FreeAndNil(Subscriber); FreeAndNil(SubscriberEventHandlers); end; procedure TSubscriberEventHandlers77.OnDataSetMessage( ASender: TObject; sender: OleVariant; const eventArgs: _EasyUADataSetMessageEventArgs); var Count: Cardinal; DictionaryEntry2: _DictionaryEntry2; Element: OleVariant; FieldDataDictionaryEnumerator: IEnumVariant; begin // Display the dataset. if eventArgs.Succeeded then begin // An event with null DataSetData just indicates a successful connection. if eventArgs.DataSetData <> nil then begin WriteLn; WriteLn('Dataset data: ', eventArgs.DataSetData.ToString); FieldDataDictionaryEnumerator := eventArgs.DataSetData.FieldDataDictionary.GetEnumerator; while (FieldDataDictionaryEnumerator.Next(1, Element, Count) = S_OK) do begin DictionaryEntry2 := IUnknown(Element) as _DictionaryEntry2; WriteLn(DictionaryEntry2.ToString); end; end; end else begin WriteLn; WriteLn('*** Failure: ', eventArgs.ErrorMessageBrief); end; end; // Example output: // //Subscribing... //Processing dataset message events for 20 seconds... // ////Dataset data: Good; Data; publisher="32", writer=1, class=eae79794-1af7-4f96-8401-4096cd1d8908, fields: 4 //[#0, True {System.Boolean} @0001-01-01T00:00:00.000 @@0001-01-01T00:00:00.000; Good] //[#1, 7945 {System.Int32} @0001-01-01T00:00:00.000 @@0001-01-01T00:00:00.000; Good] //[#2, 5246 {System.Int32} @0001-01-01T00:00:00.000 @@0001-01-01T00:00:00.000; Good] //[#3, 9/30/2019 11:19:14 AM {System.DateTime} @0001-01-01T00:00:00.000 @@0001-01-01T00:00:00.000; Good] // //Dataset data: Good; Data; publisher="32", writer=3, class=96976b7b-0db7-46c3-a715-0979884b55ae, fields: 100 //[#0, 45 {System.Int64} @0001-01-01T00:00:00.000 @@0001-01-01T00:00:00.000; Good] //[#1, 145 {System.Int64} @0001-01-01T00:00:00.000 @@0001-01-01T00:00:00.000; Good] //[#2, 245 {System.Int64} @0001-01-01T00:00:00.000 @@0001-01-01T00:00:00.000; Good] //[#3, 345 {System.Int64} @0001-01-01T00:00:00.000 @@0001-01-01T00:00:00.000; Good] //[#4, 445 {System.Int64} @0001-01-01T00:00:00.000 @@0001-01-01T00:00:00.000; Good] //[#5, 545 {System.Int64} @0001-01-01T00:00:00.000 @@0001-01-01T00:00:00.000; Good] //[#6, 645 {System.Int64} @0001-01-01T00:00:00.000 @@0001-01-01T00:00:00.000; Good] //[#7, 745 {System.Int64} @0001-01-01T00:00:00.000 @@0001-01-01T00:00:00.000; Good] //[#8, 845 {System.Int64} @0001-01-01T00:00:00.000 @@0001-01-01T00:00:00.000; Good] //[#9, 945 {System.Int64} @0001-01-01T00:00:00.000 @@0001-01-01T00:00:00.000; Good] //[#10, 1045 {System.Int64} @0001-01-01T00:00:00.000 @@0001-01-01T00:00:00.000; Good] //...
// This example shows how to subscribe to all dataset messages on an OPC-UA PubSub connection with UDP UADP mapping. // // In order to produce network messages for this example, run the UADemoPublisher tool. For documentation, see // https://kb.opclabs.com/UADemoPublisher_Basics . In some cases, you may have to specify the interface name to be used. // // Find all latest examples here: https://opclabs.doc-that.com/files/onlinedocs/OPCLabs-OpcStudio/Latest/examples.html . // OPC client and subscriber examples in PHP on GitHub: https://github.com/OPCLabs/Examples-QuickOPC-PHP . // Missing some example? Ask us for it on our Online Forums, https://www.opclabs.com/forum/index ! You do not have to own // a commercial license in order to use Online Forums, and we reply to every post. class SubscriberEvents { function DataSetMessage($Sender, $E) { // Display the dataset. if ($E->Succeeded) { // An event with null DataSetData just indicates a successful connection. printf("\n"); printf("Dataset data: %s\n", $E->DataSetData); foreach ($E->DataSetData->FieldDataDictionary as $Pair) printf("%s\n", $Pair); } else { printf("\n"); printf("*** Failure: %s\n", $E->ErrorMessageBrief); } } } // Define the PubSub connection we will work with. $SubscribeDataSetArguments = new COM("OpcLabs.EasyOpc.UA.PubSub.OperationModel.EasyUASubscribeDataSetArguments"); $ConnectionDescriptor = $SubscribeDataSetArguments->DataSetSubscriptionDescriptor->ConnectionDescriptor; $ConnectionDescriptor->ResourceAddress->ResourceDescriptor->UrlString = "opc.udp://239.0.0.1"; // In some cases you may have to set the interface (network adapter) name that needs to be used, similarly to // the statement below. Your actual interface name may differ, of course. $ConnectionDescriptor->ResourceAddress->InterfaceName = "Ethernet"; // Instantiate the subscriber object and hook events. $Subscriber = new COM("OpcLabs.EasyOpc.UA.PubSub.EasyUASubscriber"); $SubscriberEvents = new SubscriberEvents(); com_event_sink($Subscriber, $SubscriberEvents, "DEasyUASubscriberEvents"); printf("Subscribing...\n"); $Subscriber->SubscribeDataSet($SubscribeDataSetArguments); printf("Processing dataset message events for 20 seconds..."); $startTime = time(); do { com_message_pump(1000); } while (time() < $startTime + 20); printf("Unsubscribing...\n"); $Subscriber->UnsubscribeAllDataSets; printf("Waiting for 1 second..."); // Unsubscribe operation is asynchronous, messages may still come for a short while. $startTime = time(); do { com_message_pump(1000); } while (time() < $startTime + 1); printf("Finished.\n"); // Example output: // //Subscribing... //Processing dataset message events for 20 seconds... // ////Dataset data: Good; Data; publisher="32", writer=1, class=eae79794-1af7-4f96-8401-4096cd1d8908, fields: 4 //[#0, True {System.Boolean} @0001-01-01T00:00:00.000 @@0001-01-01T00:00:00.000; Good] //[#1, 7945 {System.Int32} @0001-01-01T00:00:00.000 @@0001-01-01T00:00:00.000; Good] //[#2, 5246 {System.Int32} @0001-01-01T00:00:00.000 @@0001-01-01T00:00:00.000; Good] //[#3, 9/30/2019 11:19:14 AM {System.DateTime} @0001-01-01T00:00:00.000 @@0001-01-01T00:00:00.000; Good] // //Dataset data: Good; Data; publisher="32", writer=3, class=96976b7b-0db7-46c3-a715-0979884b55ae, fields: 100 //[#0, 45 {System.Int64} @0001-01-01T00:00:00.000 @@0001-01-01T00:00:00.000; Good] //[#1, 145 {System.Int64} @0001-01-01T00:00:00.000 @@0001-01-01T00:00:00.000; Good] //[#2, 245 {System.Int64} @0001-01-01T00:00:00.000 @@0001-01-01T00:00:00.000; Good] //[#3, 345 {System.Int64} @0001-01-01T00:00:00.000 @@0001-01-01T00:00:00.000; Good] //[#4, 445 {System.Int64} @0001-01-01T00:00:00.000 @@0001-01-01T00:00:00.000; Good] //[#5, 545 {System.Int64} @0001-01-01T00:00:00.000 @@0001-01-01T00:00:00.000; Good] //[#6, 645 {System.Int64} @0001-01-01T00:00:00.000 @@0001-01-01T00:00:00.000; Good] //[#7, 745 {System.Int64} @0001-01-01T00:00:00.000 @@0001-01-01T00:00:00.000; Good] //[#8, 845 {System.Int64} @0001-01-01T00:00:00.000 @@0001-01-01T00:00:00.000; Good] //[#9, 945 {System.Int64} @0001-01-01T00:00:00.000 @@0001-01-01T00:00:00.000; Good] //[#10, 1045 {System.Int64} @0001-01-01T00:00:00.000 @@0001-01-01T00:00:00.000; Good] //...
REM This example shows how to subscribe to all dataset messages on an OPC-UA PubSub connection with UDP UADP mapping. REM REM In order to produce network messages for this example, run the UADemoPublisher tool. For documentation, see REM https://kb.opclabs.com/UADemoPublisher_Basics . In some cases, you may have to specify the interface name to be used. REM REM Find all latest examples here: https://opclabs.doc-that.com/files/onlinedocs/OPCLabs-OpcStudio/Latest/examples.html . REM OPC client and subscriber examples in Visual Basic on GitHub: https://github.com/OPCLabs/Examples-QuickOPC-VB . REM Missing some example? Ask us for it on our Online Forums, https://www.opclabs.com/forum/index ! You do not have to own REM a commercial license in order to use Online Forums, and we reply to every post. ' The subscriber object, with events 'Public WithEvents Subscriber2 As EasyUASubscriber Private Sub EasyUASubscriber_SubscribeDataSet_Main1_Command_Click() OutputText = "" ' Define the PubSub connection we will work with. Dim subscribeDataSetArguments As New EasyUASubscribeDataSetArguments Dim ConnectionDescriptor As UAPubSubConnectionDescriptor Set ConnectionDescriptor = subscribeDataSetArguments.dataSetSubscriptionDescriptor.ConnectionDescriptor ConnectionDescriptor.ResourceAddress.ResourceDescriptor.UrlString = "opc.udp://239.0.0.1" ' In some cases you may have to set the interface (network adapter) name that needs to be used, similarly to ' the statement below. Your actual interface name may differ, of course. 'ConnectionDescriptor.ResourceAddress.InterfaceName := 'Ethernet'; ' Instantiate the subscriber object and hook events. Set Subscriber2 = New EasyUASubscriber OutputText = OutputText & "Subscribing..." & vbCrLf Subscriber2.SubscribeDataSet subscribeDataSetArguments OutputText = OutputText & "Processing dataset message for 20 seconds..." & vbCrLf Pause 20000 OutputText = OutputText & "Unsubscribing..." & vbCrLf Subscriber2.UnsubscribeAllDataSets OutputText = OutputText & "Waiting for 1 second..." & vbCrLf ' Unsubscribe operation is asynchronous, messages may still come for a short while. Pause 1000 Set Subscriber2 = Nothing OutputText = OutputText & "Finished." & vbCrLf End Sub Private Sub Subscriber2_DataSetMessage(ByVal sender As Variant, ByVal eventArgs As EasyUADataSetMessageEventArgs) ' Display the dataset If eventArgs.Succeeded Then ' An event with null DataSetData just indicates a successful connection. If Not eventArgs.DataSetData Is Nothing Then OutputText = OutputText & vbCrLf OutputText = OutputText & "Dataset data: " & eventArgs.DataSetData & vbCrLf Dim dictionaryEntry2 : For Each dictionaryEntry2 In eventArgs.DataSetData.FieldDataDictionary OutputText = OutputText & dictionaryEntry2 & vbCrLf Next End If Else OutputText = OutputText & vbCrLf OutputText = OutputText & eventArgs.ErrorMessageBrief & vbCrLf End If End Sub ' Example output: ' 'Subscribing... 'Processing dataset message events for 20 seconds... ' 'Dataset data: Good; Data; publisher="32", writer=1, class=eae79794-1af7-4f96-8401-4096cd1d8908, fields: 4 '[#0, True {System.Boolean} @0001-01-01T00:00:00.000 @@0001-01-01T00:00:00.000; Good] '[#1, 7945 {System.Int32} @0001-01-01T00:00:00.000 @@0001-01-01T00:00:00.000; Good] '[#2, 5246 {System.Int32} @0001-01-01T00:00:00.000 @@0001-01-01T00:00:00.000; Good] '[#3, 9/30/2019 11:19:14 AM {System.DateTime} @0001-01-01T00:00:00.000 @@0001-01-01T00:00:00.000; Good] ' 'Dataset data: Good; Data; publisher="32", writer=3, class=96976b7b-0db7-46c3-a715-0979884b55ae, fields: 100 '[#0, 45 {System.Int64} @0001-01-01T00:00:00.000 @@0001-01-01T00:00:00.000; Good] '[#1, 145 {System.Int64} @0001-01-01T00:00:00.000 @@0001-01-01T00:00:00.000; Good] '[#2, 245 {System.Int64} @0001-01-01T00:00:00.000 @@0001-01-01T00:00:00.000; Good] '[#3, 345 {System.Int64} @0001-01-01T00:00:00.000 @@0001-01-01T00:00:00.000; Good] '[#4, 445 {System.Int64} @0001-01-01T00:00:00.000 @@0001-01-01T00:00:00.000; Good] '[#5, 545 {System.Int64} @0001-01-01T00:00:00.000 @@0001-01-01T00:00:00.000; Good] '[#6, 645 {System.Int64} @0001-01-01T00:00:00.000 @@0001-01-01T00:00:00.000; Good] '[#7, 745 {System.Int64} @0001-01-01T00:00:00.000 @@0001-01-01T00:00:00.000; Good] '[#8, 845 {System.Int64} @0001-01-01T00:00:00.000 @@0001-01-01T00:00:00.000; Good] '[#9, 945 {System.Int64} @0001-01-01T00:00:00.000 @@0001-01-01T00:00:00.000; Good] '[#10, 1045 {System.Int64} @0001-01-01T00:00:00.000 @@0001-01-01T00:00:00.000; Good] '...
Rem This example shows how to subscribe to all dataset messages on an OPC-UA PubSub connection with UDP UADP mapping. Rem Rem In order to produce network messages for this example, run the UADemoPublisher tool. For documentation, see Rem https://kb.opclabs.com/UADemoPublisher_Basics . In some cases, you may have to specify the interface name to be used. Rem Rem Find all latest examples here: https://opclabs.doc-that.com/files/onlinedocs/OPCLabs-OpcStudio/Latest/examples.html . Rem OPC client and subscriber examples in VBScript on GitHub: https://github.com/OPCLabs/Examples-QuickOPC-VBScript . Rem Missing some example? Ask us for it on our Online Forums, https://www.opclabs.com/forum/index ! You do not have to own Rem a commercial license in order to use Online Forums, and we reply to every post. Option Explicit ' Define the PubSub connection we will work with. Dim SubscribeDataSetArguments: Set SubscribeDataSetArguments = CreateObject("OpcLabs.EasyOpc.UA.PubSub.OperationModel.EasyUASubscribeDataSetArguments") Dim ConnectionDescriptor: Set ConnectionDescriptor = SubscribeDataSetArguments.DataSetSubscriptionDescriptor.ConnectionDescriptor ConnectionDescriptor.ResourceAddress.ResourceDescriptor.UrlString = "opc.udp://239.0.0.1" ' In some cases you may have to set the interface (network adapter) name that needs to be used, similarly to ' the statement below. Your actual interface name may differ, of course. ' ConnectionDescriptor.ResourceAddress.InterfaceName = "Ethernet" ' Instantiate the subscriber object and hook events. Dim Subscriber: Set Subscriber = CreateObject("OpcLabs.EasyOpc.UA.PubSub.EasyUASubscriber") WScript.ConnectObject Subscriber, "Subscriber_" WScript.Echo "Subscribing..." Subscriber.SubscribeDataSet SubscribeDataSetArguments WScript.Echo "Processing dataset message events for 20 seconds..." WScript.Sleep 20*1000 WScript.Echo "Unsubscribing..." Subscriber.UnsubscribeAllDataSets WScript.Echo "Waiting for 1 second..." ' Unsubscribe operation is asynchronous, messages may still come for a short while. WScript.Sleep 1*1000 WScript.Echo "Finished." Sub Subscriber_DataSetMessage(Sender, e) ' Display the dataset. If e.Succeeded Then ' An event with null DataSetData just indicates a successful connection. If Not (e.DataSetData Is Nothing) Then WScript.Echo WScript.Echo "Dataset data: " & e.DataSetData Dim Pair: For Each Pair in e.DataSetData.FieldDataDictionary WScript.Echo Pair Next End If Else WScript.Echo WScript.Echo "*** Failure: " & e.ErrorMessageBrief End If End Sub ' Example output: ' 'Subscribing... 'Processing dataset message events for 20 seconds... ' ''Dataset data: Good; Data; publisher="32", writer=1, class=eae79794-1af7-4f96-8401-4096cd1d8908, fields: 4 '[#0, True {System.Boolean} @0001-01-01T00:00:00.000 @@0001-01-01T00:00:00.000; Good] '[#1, 7945 {System.Int32} @0001-01-01T00:00:00.000 @@0001-01-01T00:00:00.000; Good] '[#2, 5246 {System.Int32} @0001-01-01T00:00:00.000 @@0001-01-01T00:00:00.000; Good] '[#3, 9/30/2019 11:19:14 AM {System.DateTime} @0001-01-01T00:00:00.000 @@0001-01-01T00:00:00.000; Good] ' 'Dataset data: Good; Data; publisher="32", writer=3, class=96976b7b-0db7-46c3-a715-0979884b55ae, fields: 100 '[#0, 45 {System.Int64} @0001-01-01T00:00:00.000 @@0001-01-01T00:00:00.000; Good] '[#1, 145 {System.Int64} @0001-01-01T00:00:00.000 @@0001-01-01T00:00:00.000; Good] '[#2, 245 {System.Int64} @0001-01-01T00:00:00.000 @@0001-01-01T00:00:00.000; Good] '[#3, 345 {System.Int64} @0001-01-01T00:00:00.000 @@0001-01-01T00:00:00.000; Good] '[#4, 445 {System.Int64} @0001-01-01T00:00:00.000 @@0001-01-01T00:00:00.000; Good] '[#5, 545 {System.Int64} @0001-01-01T00:00:00.000 @@0001-01-01T00:00:00.000; Good] '[#6, 645 {System.Int64} @0001-01-01T00:00:00.000 @@0001-01-01T00:00:00.000; Good] '[#7, 745 {System.Int64} @0001-01-01T00:00:00.000 @@0001-01-01T00:00:00.000; Good] '[#8, 845 {System.Int64} @0001-01-01T00:00:00.000 @@0001-01-01T00:00:00.000; Good] '[#9, 945 {System.Int64} @0001-01-01T00:00:00.000 @@0001-01-01T00:00:00.000; Good] '[#10, 1045 {System.Int64} @0001-01-01T00:00:00.000 @@0001-01-01T00:00:00.000; Good] '...
# This example shows how to subscribe to all dataset messages on an OPC-UA PubSub connection with UDP UADP mapping. # # In order to produce network messages for this example, run the UADemoPublisher tool. For documentation, see # https://kb.opclabs.com/UADemoPublisher_Basics . In some cases, you may have to specify the interface name to be used. # # Find all latest examples here: https://opclabs.doc-that.com/files/onlinedocs/OPCLabs-OpcStudio/Latest/examples.html . # OPC client and subscriber examples in Python on GitHub: https://github.com/OPCLabs/Examples-QuickOPC-Python . # Missing some example? Ask us for it on our Online Forums, https://www.opclabs.com/forum/index ! You do not have to own # a commercial license in order to use Online Forums, and we reply to every post. # The QuickOPC package is needed. Install it using "pip install opclabs_quickopc". import opclabs_quickopc import time # Import .NET namespaces. from OpcLabs.EasyOpc.UA.PubSub import * from OpcLabs.EasyOpc.UA.PubSub.OperationModel import * def dataSetMessage(sender, e): # Display the dataset. if e.Succeeded: # An event with null DataSetData just indicates a successful connection. if e.DataSetData is not None: print('') print('Dataset data: ', e.DataSetData, sep='') for pair in e.DataSetData.FieldDataDictionary: print(pair) else: print('') print('*** Failure: ', e.ErrorMessageBrief, sep='') # Define the PubSub connection we will work with. Uses implicit conversion from a string. pubSubConnectionDescriptor = UAPubSubConnectionDescriptor.op_Implicit('opc.udp://239.0.0.1') # In some cases you may have to set the interface (network adapter) name that needs to be used, similarly to # the statement below. Your actual interface name may differ, of course. #pubSubConnectionDescriptor.ResourceAddress.InterfaceName = 'Ethernet' # Instantiate the subscriber object and hook events. subscriber = EasyUASubscriber() subscriber.DataSetMessage += dataSetMessage print('Subscribing...') IEasyUASubscriberExtension.SubscribeDataSet(subscriber, pubSubConnectionDescriptor) print('Processing dataset message events for 20 seconds...') time.sleep(20) print('Unsubscribing...') subscriber.UnsubscribeAllDataSets() print('Waiting for 1 second...') # Unsubscribe operation is asynchronous, messages may still come for a short while. time.sleep(1) subscriber.DataSetMessage -= dataSetMessage print('Finished.') # Example output: # #Subscribing... #Processing dataset message events for 20 seconds... # ##Dataset data: Good; Data; publisher="32", writer=1, class=eae79794-1af7-4f96-8401-4096cd1d8908, fields: 4 #[#0, True {System.Boolean} @0001-01-01T00:00:00.000 @@0001-01-01T00:00:00.000; Good] #[#1, 7945 {System.Int32} @0001-01-01T00:00:00.000 @@0001-01-01T00:00:00.000; Good] #[#2, 5246 {System.Int32} @0001-01-01T00:00:00.000 @@0001-01-01T00:00:00.000; Good] #[#3, 9/30/2019 11:19:14 AM {System.DateTime} @0001-01-01T00:00:00.000 @@0001-01-01T00:00:00.000; Good] # #Dataset data: Good; Data; publisher="32", writer=3, class=96976b7b-0db7-46c3-a715-0979884b55ae, fields: 100 #[#0, 45 {System.Int64} @0001-01-01T00:00:00.000 @@0001-01-01T00:00:00.000; Good] #[#1, 145 {System.Int64} @0001-01-01T00:00:00.000 @@0001-01-01T00:00:00.000; Good] #[#2, 245 {System.Int64} @0001-01-01T00:00:00.000 @@0001-01-01T00:00:00.000; Good] #[#3, 345 {System.Int64} @0001-01-01T00:00:00.000 @@0001-01-01T00:00:00.000; Good] #[#4, 445 {System.Int64} @0001-01-01T00:00:00.000 @@0001-01-01T00:00:00.000; Good] #[#5, 545 {System.Int64} @0001-01-01T00:00:00.000 @@0001-01-01T00:00:00.000; Good] #[#6, 645 {System.Int64} @0001-01-01T00:00:00.000 @@0001-01-01T00:00:00.000; Good] #[#7, 745 {System.Int64} @0001-01-01T00:00:00.000 @@0001-01-01T00:00:00.000; Good] #[#8, 845 {System.Int64} @0001-01-01T00:00:00.000 @@0001-01-01T00:00:00.000; Good] #[#9, 945 {System.Int64} @0001-01-01T00:00:00.000 @@0001-01-01T00:00:00.000; Good] #[#10, 1045 {System.Int64} @0001-01-01T00:00:00.000 @@0001-01-01T00:00:00.000; Good] #...
# This example shows how to subscribe to all dataset messages on an OPC-UA PubSub connection, and pull events, and display # the incoming datasets. # # In order to produce network messages for this example, run the UADemoPublisher tool. For documentation, see # https://kb.opclabs.com/UADemoPublisher_Basics . In some cases, you may have to specify the interface name to be used. # # The Python for Windows (pywin32) extensions package is needed. Install it using "pip install pypiwin32". # CAUTION: We now recommend using Python.NET package instead. Full set of examples with Python.NET is available! # # Find all latest examples here: https://opclabs.doc-that.com/files/onlinedocs/OPCLabs-OpcStudio/Latest/examples.html . import time import win32com.client # Define the PubSub connection we will work with. subscribeDataSetArguments = win32com.client.Dispatch('OpcLabs.EasyOpc.UA.PubSub.OperationModel.EasyUASubscribeDataSetArguments') connectionDescriptor = subscribeDataSetArguments.DataSetSubscriptionDescriptor.ConnectionDescriptor connectionDescriptor.ResourceAddress.ResourceDescriptor.UrlString = 'opc.udp://239.0.0.1' # In some cases you may have to set the interface (network adapter) name that needs to be used, similarly to # the statement below. Your actual interface name may differ, of course. #connectionDescriptor.ResourceAddress.InterfaceName = 'Ethernet' # Instantiate the subscriber object. subscriber = win32com.client.Dispatch('OpcLabs.EasyOpc.UA.PubSub.EasyUASubscriber') # In order to use event pull, you must set a non-zero queue capacity upfront. subscriber.PullDataSetMessageQueueCapacity = 1000 print('Subscribing...') subscriber.SubscribeDataSet(subscribeDataSetArguments) print('Processing dataset message events for 20 seconds...') endTime = time.time() + 20 while time.time() < endTime: eventArgs = subscriber.PullDataSetMessage(2*1000) if eventArgs is not None: # Display the dataset. if eventArgs.Succeeded: # An event with null DataSetData just indicates a successful connection. if eventArgs.DataSetData is not None: print('') print('Dataset data: ', eventArgs.DataSetData) for pair in eventArgs.DataSetData.FieldDataDictionary: print(pair) else: print('') print('*** Failure: ', eventArgs.ErrorMessageBrief) print('Unsubscribing...') subscriber.UnsubscribeAllDataSets print('Waiting for 1 second...') # Unsubscribe operation is asynchronous, messages may still come for a short while. endTime = time.time() + 1 while time.time() < endTime: pass print('Finished.') # Example output: # #Subscribing... #Processing dataset message events for 20 seconds... # ##Dataset data: Good; Data; publisher="32", writer=1, class=eae79794-1af7-4f96-8401-4096cd1d8908, fields: 4 #[#0, True {System.Boolean} @0001-01-01T00:00:00.000 @@0001-01-01T00:00:00.000; Good] #[#1, 7945 {System.Int32} @0001-01-01T00:00:00.000 @@0001-01-01T00:00:00.000; Good] #[#2, 5246 {System.Int32} @0001-01-01T00:00:00.000 @@0001-01-01T00:00:00.000; Good] #[#3, 9/30/2019 11:19:14 AM {System.DateTime} @0001-01-01T00:00:00.000 @@0001-01-01T00:00:00.000; Good] # #Dataset data: Good; Data; publisher="32", writer=3, class=96976b7b-0db7-46c3-a715-0979884b55ae, fields: 100 #[#0, 45 {System.Int64} @0001-01-01T00:00:00.000 @@0001-01-01T00:00:00.000; Good] #[#1, 145 {System.Int64} @0001-01-01T00:00:00.000 @@0001-01-01T00:00:00.000; Good] #[#2, 245 {System.Int64} @0001-01-01T00:00:00.000 @@0001-01-01T00:00:00.000; Good] #[#3, 345 {System.Int64} @0001-01-01T00:00:00.000 @@0001-01-01T00:00:00.000; Good] #[#4, 445 {System.Int64} @0001-01-01T00:00:00.000 @@0001-01-01T00:00:00.000; Good] #[#5, 545 {System.Int64} @0001-01-01T00:00:00.000 @@0001-01-01T00:00:00.000; Good] #[#6, 645 {System.Int64} @0001-01-01T00:00:00.000 @@0001-01-01T00:00:00.000; Good] #[#7, 745 {System.Int64} @0001-01-01T00:00:00.000 @@0001-01-01T00:00:00.000; Good] #[#8, 845 {System.Int64} @0001-01-01T00:00:00.000 @@0001-01-01T00:00:00.000; Good] #[#9, 945 {System.Int64} @0001-01-01T00:00:00.000 @@0001-01-01T00:00:00.000; Good] #[#10, 1045 {System.Int64} @0001-01-01T00:00:00.000 @@0001-01-01T00:00:00.000; Good] #...
# This example shows how to subscribe to all dataset messages on an OPC-UA PubSub connection, and pull events, and # display the incoming datasets. # # In order to produce network messages for this example, run the UADemoPublisher tool. For documentation, see # https://kb.opclabs.com/UADemoPublisher_Basics . In some cases, you may have to specify the interface name to be used. # # Find all latest examples here: https://opclabs.doc-that.com/files/onlinedocs/OPCLabs-OpcStudio/Latest/examples.html . # OPC client and subscriber examples in Python on GitHub: https://github.com/OPCLabs/Examples-QuickOPC-Python . # Missing some example? Ask us for it on our Online Forums, https://www.opclabs.com/forum/index ! You do not have to own # a commercial license in order to use Online Forums, and we reply to every post. # The QuickOPC package is needed. Install it using "pip install opclabs_quickopc". import opclabs_quickopc import time # Import .NET namespaces. from OpcLabs.EasyOpc.UA.PubSub import * from OpcLabs.EasyOpc.UA.PubSub.OperationModel import * # Define the PubSub connection we will work with. Uses implicit conversion from a string. pubSubConnectionDescriptor = UAPubSubConnectionDescriptor.op_Implicit('opc.udp://239.0.0.1') # In some cases you may have to set the interface (network adapter) name that needs to be used, similarly to # the statement below. Your actual interface name may differ, of course. #pubSubConnectionDescriptor.ResourceAddress.InterfaceName = 'Ethernet' # Instantiate the subscriber object. subscriber = EasyUASubscriber() # In order to use event pull, you must set a non-zero queue capacity upfront. subscriber.PullDataSetMessageQueueCapacity = 1000 print('Subscribing...') IEasyUASubscriberExtension.SubscribeDataSet(subscriber, pubSubConnectionDescriptor) print('Processing dataset message events for 20 seconds...') endTime = time.time() + 20 while time.time() < endTime: eventArgs = IEasyUASubscriberExtension.PullDataSetMessage(subscriber, 2*1000) if eventArgs is not None: # Display the dataset. if eventArgs.Succeeded: # An event with null DataSetData just indicates a successful connection. if eventArgs.DataSetData is not None: print('') print('Dataset data: ', eventArgs.DataSetData, sep='') for pair in eventArgs.DataSetData.FieldDataDictionary: print(pair) else: print('') print('*** Failure: ', eventArgs.ErrorMessageBrief, sep='') print('Unsubscribing...') subscriber.UnsubscribeAllDataSets() print('Waiting for 1 second...') # Unsubscribe operation is asynchronous, messages may still come for a short while. time.sleep(1) print('Finished.') # Example output: # #Subscribing... #Processing dataset message events for 20 seconds... # ##Dataset data: Good; Data; publisher="32", writer=1, class=eae79794-1af7-4f96-8401-4096cd1d8908, fields: 4 #[#0, True {System.Boolean} @0001-01-01T00:00:00.000 @@0001-01-01T00:00:00.000; Good] #[#1, 7945 {System.Int32} @0001-01-01T00:00:00.000 @@0001-01-01T00:00:00.000; Good] #[#2, 5246 {System.Int32} @0001-01-01T00:00:00.000 @@0001-01-01T00:00:00.000; Good] #[#3, 9/30/2019 11:19:14 AM {System.DateTime} @0001-01-01T00:00:00.000 @@0001-01-01T00:00:00.000; Good] # #Dataset data: Good; Data; publisher="32", writer=3, class=96976b7b-0db7-46c3-a715-0979884b55ae, fields: 100 #[#0, 45 {System.Int64} @0001-01-01T00:00:00.000 @@0001-01-01T00:00:00.000; Good] #[#1, 145 {System.Int64} @0001-01-01T00:00:00.000 @@0001-01-01T00:00:00.000; Good] #[#2, 245 {System.Int64} @0001-01-01T00:00:00.000 @@0001-01-01T00:00:00.000; Good] #[#3, 345 {System.Int64} @0001-01-01T00:00:00.000 @@0001-01-01T00:00:00.000; Good] #[#4, 445 {System.Int64} @0001-01-01T00:00:00.000 @@0001-01-01T00:00:00.000; Good] #[#5, 545 {System.Int64} @0001-01-01T00:00:00.000 @@0001-01-01T00:00:00.000; Good] #[#6, 645 {System.Int64} @0001-01-01T00:00:00.000 @@0001-01-01T00:00:00.000; Good] #[#7, 745 {System.Int64} @0001-01-01T00:00:00.000 @@0001-01-01T00:00:00.000; Good] #[#8, 845 {System.Int64} @0001-01-01T00:00:00.000 @@0001-01-01T00:00:00.000; Good] #[#9, 945 {System.Int64} @0001-01-01T00:00:00.000 @@0001-01-01T00:00:00.000; Good] #[#10, 1045 {System.Int64} @0001-01-01T00:00:00.000 @@0001-01-01T00:00:00.000; Good] #...
System.Object
System.EventArgs
OpcLabs.BaseLib.OperationModel.OperationEventArgs
OpcLabs.EasyOpc.UA.PubSub.OperationModel.EasyUAPubSubMessageEventArgs
OpcLabs.EasyOpc.UA.PubSub.OperationModel.EasyUADataSetMessageEventArgs