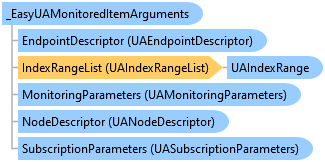
'Declaration
<CLSCompliantAttribute(False)> <ComVisibleAttribute(True)> <GuidAttribute("751F09D0-7C37-4E42-8719-26471E33010B")> <InterfaceTypeAttribute(ComInterfaceType.InterfaceIsDual)> Public Interface _EasyUAMonitoredItemArguments
'Usage
Dim instance As _EasyUAMonitoredItemArguments
[CLSCompliant(false)] [ComVisible(true)] [Guid("751F09D0-7C37-4E42-8719-26471E33010B")] [InterfaceType(ComInterfaceType.InterfaceIsDual)] public interface _EasyUAMonitoredItemArguments
[CLSCompliant(false)] [ComVisible(true)] [Guid("751F09D0-7C37-4E42-8719-26471E33010B")] [InterfaceType(ComInterfaceType.InterfaceIsDual)] public interface class _EasyUAMonitoredItemArguments
This member or type is for use from COM. It is not meant to be used from .NET or Python. Refer to the corresponding .NET member or type instead, if you are developing in .NET or Python.
// This example shows how to subscribe to changes of multiple monitored items and display the value of the monitored item with // each change. // // Find all latest examples here: https://opclabs.doc-that.com/files/onlinedocs/OPCLabs-OpcStudio/Latest/examples.html . // Missing some example? Ask us for it on our Online Forums, https://www.opclabs.com/forum/index ! You do not have to own // a commercial license in order to use Online Forums, and we reply to every post. #include "stdafx.h" // Includes "QuickOpc.h", and other commonly used files #include <atlcom.h> #include <atlsafe.h> #include "SubscribeMultipleMonitoredItems.h" namespace _EasyUAClient { // CEasyUAClientEvents class CEasyUAClientEvents : public IDispEventImpl<1, CEasyUAClientEvents> { public: BEGIN_SINK_MAP(CEasyUAClientEvents) // Event handlers must have the __stdcall calling convention SINK_ENTRY(1, DISPID_EASYUACLIENTEVENTS_DATACHANGENOTIFICATION, &CEasyUAClientEvents::DataChangeNotification) END_SINK_MAP() public: // The handler for EasyUAClient.DataChangeNotification event STDMETHOD(DataChangeNotification)(VARIANT varSender, _EasyUADataChangeNotificationEventArgs* pEventArgs) { // Display the data _tprintf(_T("%s: "), (LPCTSTR)CW2CT(pEventArgs->Arguments->NodeDescriptor->ToString)); // Remark: Production code would check EventArgsPtr->Exception before accessing EventArgsPtr->AttributeData. _UAAttributeDataPtr AttributeDataPtr(pEventArgs->AttributeData); _tprintf(_T("%s\n"), (LPCTSTR)CW2CT(AttributeDataPtr->ToString)); return S_OK; } }; void SubscribeMultipleMonitoredItems::Main() { // Initialize the COM library CoInitializeEx(NULL, COINIT_MULTITHREADED); { // Instantiate the client object _EasyUAClientPtr ClientPtr(__uuidof(EasyUAClient)); // Hook events CEasyUAClientEvents* pClientEvents = new CEasyUAClientEvents(); AtlGetObjectSourceInterface(ClientPtr, &pClientEvents->m_libid, &pClientEvents->m_iid, &pClientEvents->m_wMajorVerNum, &pClientEvents->m_wMinorVerNum); pClientEvents->m_iid = _uuidof(DEasyUAClientEvents); pClientEvents->DispEventAdvise(ClientPtr, &pClientEvents->m_iid); // _tprintf(_T("Subscribing...\n")); _UAMonitoringParametersPtr MonitoringParametersPtr(_uuidof(UAMonitoringParameters)); MonitoringParametersPtr->SamplingInterval = 1000; _UAMonitoredItemArgumentsPtr MonitoringArguments1Ptr(_uuidof(EasyUAMonitoredItemArguments)); MonitoringArguments1Ptr->EndpointDescriptor->UrlString = //L"http://opcua.demo-this.com:51211/UA/SampleServer"; L"opc.tcp://opcua.demo-this.com:51210/UA/SampleServer"; MonitoringArguments1Ptr->NodeDescriptor->NodeId->ExpandedText = L"nsu=http://test.org/UA/Data/ ;i=10845"; MonitoringArguments1Ptr->MonitoringParameters = MonitoringParametersPtr; _UAMonitoredItemArgumentsPtr MonitoringArguments2Ptr(_uuidof(EasyUAMonitoredItemArguments)); MonitoringArguments2Ptr->EndpointDescriptor->UrlString = //L"http://opcua.demo-this.com:51211/UA/SampleServer"; L"opc.tcp://opcua.demo-this.com:51210/UA/SampleServer"; MonitoringArguments2Ptr->NodeDescriptor->NodeId->ExpandedText = L"nsu=http://test.org/UA/Data/ ;i=10853"; MonitoringArguments2Ptr->MonitoringParameters = MonitoringParametersPtr; _UAMonitoredItemArgumentsPtr MonitoringArguments3Ptr(_uuidof(EasyUAMonitoredItemArguments)); MonitoringArguments3Ptr->EndpointDescriptor->UrlString = //L"http://opcua.demo-this.com:51211/UA/SampleServer"; L"opc.tcp://opcua.demo-this.com:51210/UA/SampleServer"; MonitoringArguments3Ptr->NodeDescriptor->NodeId->ExpandedText = L"nsu=http://test.org/UA/Data/ ;i=10855"; MonitoringArguments3Ptr->MonitoringParameters = MonitoringParametersPtr; CComSafeArray<VARIANT> arguments(3); arguments.SetAt(0, _variant_t((IDispatch*)MonitoringArguments1Ptr)); arguments.SetAt(1, _variant_t((IDispatch*)MonitoringArguments2Ptr)); arguments.SetAt(2, _variant_t((IDispatch*)MonitoringArguments3Ptr)); CComVariant vArguments(arguments); CComSafeArray<VARIANT> handles; handles.Attach(ClientPtr->SubscribeMultipleMonitoredItems(&vArguments)); for (int i = handles.GetLowerBound(); i <= handles.GetUpperBound(); i++) { _variant_t vString; vString.ChangeType(VT_BSTR, &_variant_t(handles[i])); _tprintf(_T("handleArray(d)s\n"), i, (LPCTSTR)CW2CT((_bstr_t)vString)); } _tprintf(_T("Processing monitored item changed events for 10 seconds...\n")); Sleep(10*1000); _tprintf(_T("Unsubscribing...\n")); ClientPtr->UnsubscribeAllMonitoredItems(); _tprintf(_T("Waiting for 5 seconds...\n")); Sleep(5*1000); // Unhook events pClientEvents->DispEventUnadvise(ClientPtr, &pClientEvents->m_iid); } // Release all interface pointers BEFORE calling CoUninitialize() CoUninitialize(); } }
// This example shows how to subscribe to changes of multiple monitored items and display the value of the monitored item with // each change. // // Find all latest examples here: https://opclabs.doc-that.com/files/onlinedocs/OPCLabs-OpcStudio/Latest/examples.html . // Missing some example? Ask us for it on our Online Forums, https://www.opclabs.com/forum/index ! You do not have to own // a commercial license in order to use Online Forums, and we reply to every post. { type TClientEventHandlers = class procedure OnDataChangeNotification( Sender: TObject; sender0: OleVariant; eventArgs: _EasyUADataChangeNotificationEventArgs); end; procedure TClientEventHandlers.OnDataChangeNotification( Sender: TObject; sender0: OleVariant; eventArgs: _EasyUADataChangeNotificationEventArgs); begin // Display the data // Remark: Production code would check eventArgs.Exception before accessing // eventArgs.AttributeData. WriteLn(eventArgs.Arguments.NodeDescriptor.ToString, ': ', eventArgs.AttributeData.ToString); end; } class procedure SubscribeMultipleMonitoredItems.Main; var Arguments: OleVariant; EvsClient: TEvsEasyUAClient; Client: EasyUAClient; ClientEventHandlers: TClientEventHandlers; Handle: Cardinal; HandleArray: OleVariant; I: Cardinal; MonitoredItemArguments1, MonitoredItemArguments2, MonitoredItemArguments3: _EasyUAMonitoredItemArguments; MonitoringParameters: _UAMonitoringParameters; begin // Instantiate the client object and hook events EvsClient := TEvsEasyUAClient.Create(nil); Client := EvsClient.ComServer; ClientEventHandlers := TClientEventHandlers.Create; EvsClient.OnDataChangeNotification := @ClientEventHandlers.OnDataChangeNotification; WriteLn('Subscribing...'); MonitoringParameters := CoUAMonitoringParameters.Create; MonitoringParameters.SamplingInterval := 1000; MonitoredItemArguments1 := CoEasyUAMonitoredItemArguments.Create; MonitoredItemArguments1.EndpointDescriptor.UrlString := //'http://opcua.demo-this.com:51211/UA/SampleServer'; 'opc.tcp://opcua.demo-this.com:51210/UA/SampleServer'; MonitoredItemArguments1.NodeDescriptor.NodeId.ExpandedText := 'nsu=http://test.org/UA/Data/ ;i=10845'; MonitoredItemArguments1.MonitoringParameters := MonitoringParameters; MonitoredItemArguments2 := CoEasyUAMonitoredItemArguments.Create; MonitoredItemArguments2.EndpointDescriptor.UrlString := //'http://opcua.demo-this.com:51211/UA/SampleServer'; 'opc.tcp://opcua.demo-this.com:51210/UA/SampleServer'; MonitoredItemArguments2.NodeDescriptor.NodeId.ExpandedText := 'nsu=http://test.org/UA/Data/ ;i=10853'; MonitoredItemArguments2.MonitoringParameters := MonitoringParameters; MonitoredItemArguments3 := CoEasyUAMonitoredItemArguments.Create; MonitoredItemArguments3.EndpointDescriptor.UrlString := //'http://opcua.demo-this.com:51211/UA/SampleServer'; 'opc.tcp://opcua.demo-this.com:51210/UA/SampleServer'; MonitoredItemArguments3.NodeDescriptor.NodeId.ExpandedText := 'nsu=http://test.org/UA/Data/ ;i=10855'; MonitoredItemArguments3.MonitoringParameters := MonitoringParameters; Arguments := VarArrayCreate([0, 2], varVariant); Arguments[0] := MonitoredItemArguments1; Arguments[1] := MonitoredItemArguments2; Arguments[2] := MonitoredItemArguments3; TVarData(HandleArray).VType := varArray or varVariant; TVarData(HandleArray).VArray := PVarArray( Client.SubscribeMultipleMonitoredItems(PSafeArray(TVarData(Arguments).VArray))); for I := VarArrayLowBound(HandleArray, 1) to VarArrayHighBound(HandleArray, 1) do begin Handle := Cardinal(HandleArray[I]); WriteLn('HandleArray[', I, ']: ', Handle); end; WriteLn('Processing monitored item changed events for 10 seconds...'); PumpSleep(10*1000); WriteLn('Unsubscribing...'); Client.UnsubscribeAllMonitoredItems; WriteLn('Waiting for 5 seconds...'); Sleep(5*1000); WriteLn('Finished...'); end;
// This example shows how to subscribe to changes of multiple monitored items and display the value of the monitored item with // each change. // // Find all latest examples here: https://opclabs.doc-that.com/files/onlinedocs/OPCLabs-OpcStudio/Latest/examples.html . // OPC client and subscriber examples in Object Pascal (Delphi) on GitHub: https://github.com/OPCLabs/Examples-QuickOPC-OP . // Missing some example? Ask us for it on our Online Forums, https://www.opclabs.com/forum/index ! You do not have to own // a commercial license in order to use Online Forums, and we reply to every post. type TClientEventHandlers123 = class procedure OnDataChangeNotification( ASender: TObject; sender: OleVariant; const eventArgs: _EasyUADataChangeNotificationEventArgs); end; procedure TClientEventHandlers123.OnDataChangeNotification( ASender: TObject; sender: OleVariant; const eventArgs: _EasyUADataChangeNotificationEventArgs); begin // Display the data if eventArgs.Succeeded then WriteLn(eventArgs.Arguments.NodeDescriptor.ToString, ': ', eventArgs.AttributeData.ToString) else WriteLn(eventArgs.Arguments.NodeDescriptor.ToString, ' *** Failure: ', eventArgs.ErrorMessageBrief); end; class procedure SubscribeMultipleMonitoredItems.Main; var Arguments: OleVariant; Client: TEasyUAClient; ClientEventHandlers: TClientEventHandlers123; Handle: Cardinal; HandleArray: OleVariant; I: Cardinal; MonitoredItemArguments1, MonitoredItemArguments2, MonitoredItemArguments3: _EasyUAMonitoredItemArguments; MonitoringParameters: _UAMonitoringParameters; begin // Instantiate the client object and hook events Client := TEasyUAClient.Create(nil); ClientEventHandlers := TClientEventHandlers123.Create; Client.OnDataChangeNotification := ClientEventHandlers.OnDataChangeNotification; WriteLn('Subscribing...'); MonitoringParameters := CoUAMonitoringParameters.Create; MonitoringParameters.SamplingInterval := 1000; MonitoredItemArguments1 := CoEasyUAMonitoredItemArguments.Create; MonitoredItemArguments1.EndpointDescriptor.UrlString := //'http://opcua.demo-this.com:51211/UA/SampleServer'; //'https://opcua.demo-this.com:51212/UA/SampleServer/'; 'opc.tcp://opcua.demo-this.com:51210/UA/SampleServer'; MonitoredItemArguments1.NodeDescriptor.NodeId.ExpandedText := 'nsu=http://test.org/UA/Data/ ;i=10845'; MonitoredItemArguments1.MonitoringParameters := MonitoringParameters; MonitoredItemArguments2 := CoEasyUAMonitoredItemArguments.Create; MonitoredItemArguments2.EndpointDescriptor.UrlString := //'http://opcua.demo-this.com:51211/UA/SampleServer'; //'https://opcua.demo-this.com:51212/UA/SampleServer/'; 'opc.tcp://opcua.demo-this.com:51210/UA/SampleServer'; MonitoredItemArguments2.NodeDescriptor.NodeId.ExpandedText := 'nsu=http://test.org/UA/Data/ ;i=10853'; MonitoredItemArguments2.MonitoringParameters := MonitoringParameters; MonitoredItemArguments3 := CoEasyUAMonitoredItemArguments.Create; MonitoredItemArguments3.EndpointDescriptor.UrlString := //'http://opcua.demo-this.com:51211/UA/SampleServer'; //'https://opcua.demo-this.com:51212/UA/SampleServer/'; 'opc.tcp://opcua.demo-this.com:51210/UA/SampleServer'; MonitoredItemArguments3.NodeDescriptor.NodeId.ExpandedText := 'nsu=http://test.org/UA/Data/ ;i=10855'; MonitoredItemArguments3.MonitoringParameters := MonitoringParameters; Arguments := VarArrayCreate([0, 2], varVariant); Arguments[0] := MonitoredItemArguments1; Arguments[1] := MonitoredItemArguments2; Arguments[2] := MonitoredItemArguments3; TVarData(HandleArray).VType := varArray or varVariant; TVarData(HandleArray).VArray := PVarArray( Client.SubscribeMultipleMonitoredItems(Arguments)); for I := VarArrayLowBound(HandleArray, 1) to VarArrayHighBound(HandleArray, 1) do begin Handle := Cardinal(HandleArray[I]); WriteLn('HandleArray[', I, ']: ', Handle); end; WriteLn('Processing monitored item changed events for 10 seconds...'); PumpSleep(10*1000); WriteLn('Unsubscribing...'); Client.UnsubscribeAllMonitoredItems; WriteLn('Waiting for 5 seconds...'); Sleep(5*1000); WriteLn('Finished.'); VarClear(HandleArray); VarClear(Arguments); FreeAndNil(Client); FreeAndNil(ClientEventHandlers); end;
// This example shows how to subscribe to changes of multiple monitored items and display the value of the monitored item with // each change. // // Find all latest examples here: https://opclabs.doc-that.com/files/onlinedocs/OPCLabs-OpcStudio/Latest/examples.html . // OPC client and subscriber examples in PHP on GitHub: https://github.com/OPCLabs/Examples-QuickOPC-PHP . // Missing some example? Ask us for it on our Online Forums, https://www.opclabs.com/forum/index ! You do not have to own // a commercial license in order to use Online Forums, and we reply to every post. class ClientEvents { function DataChangeNotification($Sender, $E) { // Display the data if ($E->Succeeded) printf(" s\n", $E->Arguments->NodeDescriptor, $E->AttributeData); else printf("s *** Failures\n", $E->Arguments->NodeDescriptor, $E->ErrorMessageBrief); } } // Instantiate the client object and hook events $Client = new COM("OpcLabs.EasyOpc.UA.EasyUAClient"); $ClientEvents = new ClientEvents(); com_event_sink($Client, $ClientEvents, "DEasyUAClientEvents"); printf("Subscribing...\n"); $MonitoringParameters = new COM("OpcLabs.EasyOpc.UA.UAMonitoringParameters"); $MonitoringParameters->SamplingInterval = 1000; $MonitoredItemArguments1 = new COM("OpcLabs.EasyOpc.UA.OperationModel.EasyUAMonitoredItemArguments"); $MonitoredItemArguments1->EndpointDescriptor->UrlString = //"http://opcua.demo-this.com:51211/UA/SampleServer"; //"https://opcua.demo-this.com:51212/UA/SampleServer/"; "opc.tcp://opcua.demo-this.com:51210/UA/SampleServer"; $MonitoredItemArguments1->NodeDescriptor->NodeId->ExpandedText = "nsu=http://test.org/UA/Data/ ;i=10845"; $MonitoredItemArguments1->MonitoringParameters = $MonitoringParameters; $MonitoredItemArguments2 = new COM("OpcLabs.EasyOpc.UA.OperationModel.EasyUAMonitoredItemArguments"); $MonitoredItemArguments2->EndpointDescriptor->UrlString = //"http://opcua.demo-this.com:51211/UA/SampleServer"; //"https://opcua.demo-this.com:51212/UA/SampleServer/"; "opc.tcp://opcua.demo-this.com:51210/UA/SampleServer"; $MonitoredItemArguments2->NodeDescriptor->NodeId->ExpandedText = "nsu=http://test.org/UA/Data/ ;i=10853"; $MonitoredItemArguments2->MonitoringParameters = $MonitoringParameters; $MonitoredItemArguments3 = new COM("OpcLabs.EasyOpc.UA.OperationModel.EasyUAMonitoredItemArguments"); $MonitoredItemArguments3->EndpointDescriptor->UrlString = //"http://opcua.demo-this.com:51211/UA/SampleServer"; //"https://opcua.demo-this.com:51212/UA/SampleServer/"; "opc.tcp://opcua.demo-this.com:51210/UA/SampleServer"; $MonitoredItemArguments3->NodeDescriptor->NodeId->ExpandedText = "nsu=http://test.org/UA/Data/ ;i=10855"; $MonitoredItemArguments3->MonitoringParameters = $MonitoringParameters; $arguments[0] = $MonitoredItemArguments1; $arguments[1] = $MonitoredItemArguments2; $arguments[2] = $MonitoredItemArguments3; $handleArray = $Client->SubscribeMultipleMonitoredItems($arguments); for ($i = 0; $i < count($handleArray); $i++) { printf("handleArray[d]d\n", $i, $handleArray[$i]); } printf("Processing monitored item changed events for 10 seconds...\n"); $startTime = time(); do { com_message_pump(1000); } while (time() < $startTime + 10); printf("Unsubscribing...\n"); $Client->UnsubscribeAllMonitoredItems; printf("Waiting for 5 seconds...\n"); $startTime = time(); do { com_message_pump(1000); } while (time() < $startTime + 5);
REM This example shows how to subscribe to changes of multiple monitored items and display the value of the monitored item with REM each change. REM REM Find all latest examples here: https://opclabs.doc-that.com/files/onlinedocs/OPCLabs-OpcStudio/Latest/examples.html . REM OPC client and subscriber examples in Visual Basic on GitHub: https://github.com/OPCLabs/Examples-QuickOPC-VB . REM Missing some example? Ask us for it on our Online Forums, https://www.opclabs.com/forum/index ! You do not have to own REM a commercial license in order to use Online Forums, and we reply to every post. ' The client object, with events 'Public WithEvents Client2 As EasyUAClient Public Sub SubscribeMultipleMonitoredItems_Main_Command_Click() OutputText = "" Set Client2 = New EasyUAClient OutputText = OutputText & "Subscribing..." & vbCrLf Dim MonitoringParameters As New UAMonitoringParameters MonitoringParameters.SamplingInterval = 1000 Dim MonitoredItemArguments1 As New EasyUAMonitoredItemArguments MonitoredItemArguments1.endpointDescriptor.UrlString = "opc.tcp://opcua.demo-this.com:51210/UA/SampleServer" MonitoredItemArguments1.nodeDescriptor.NodeId.expandedText = "nsu=http://test.org/UA/Data/ ;i=10845" Set MonitoredItemArguments1.MonitoringParameters = MonitoringParameters MonitoredItemArguments1.SetState ("Item1") Dim MonitoredItemArguments2 As New EasyUAMonitoredItemArguments MonitoredItemArguments2.endpointDescriptor.UrlString = "opc.tcp://opcua.demo-this.com:51210/UA/SampleServer" MonitoredItemArguments2.nodeDescriptor.NodeId.expandedText = "nsu=http://test.org/UA/Data/ ;i=10853" Set MonitoredItemArguments2.MonitoringParameters = MonitoringParameters MonitoredItemArguments2.SetState ("Item2") Dim MonitoredItemArguments3 As New EasyUAMonitoredItemArguments MonitoredItemArguments3.endpointDescriptor.UrlString = "opc.tcp://opcua.demo-this.com:51210/UA/SampleServer" MonitoredItemArguments3.nodeDescriptor.NodeId.expandedText = "nsu=http://test.org/UA/Data/ ;i=10855" Set MonitoredItemArguments3.MonitoringParameters = MonitoringParameters MonitoredItemArguments3.SetState ("Item3") Dim arguments(2) As Variant Set arguments(0) = MonitoredItemArguments1 Set arguments(1) = MonitoredItemArguments2 Set arguments(2) = MonitoredItemArguments3 Dim handleArray As Variant handleArray = Client2.SubscribeMultipleMonitoredItems(arguments) Dim i As Long: For i = LBound(handleArray) To UBound(handleArray) OutputText = OutputText & "handleArray(" & i & "): " & handleArray(i) & vbCrLf Next OutputText = OutputText & "Processing monitored item changed events for 10 seconds..." & vbCrLf Pause 10000 OutputText = OutputText & "Unsubscribing..." & vbCrLf Call Client2.UnsubscribeAllMonitoredItems OutputText = OutputText & "Waiting for 5 seconds..." & vbCrLf Pause 5000 Set Client2 = Nothing OutputText = OutputText & "Finished." & vbCrLf End Sub Public Sub Client2_DataChangeNotification(ByVal sender As Variant, ByVal eventArgs As EasyUADataChangeNotificationEventArgs) ' Display the data If eventArgs.Exception Is Nothing Then OutputText = OutputText & "[" & eventArgs.arguments.State & "] " & eventArgs.arguments.nodeDescriptor & ": " & eventArgs.AttributeData & vbCrLf Else OutputText = OutputText & "[" & eventArgs.arguments.State & "] " & eventArgs.arguments.nodeDescriptor & ": " & eventArgs.ErrorMessageBrief & vbCrLf End If End Sub
Rem This example shows how to subscribe to changes of multiple monitored items and display the value of the monitored item with Rem each change. Rem Rem Find all latest examples here: https://opclabs.doc-that.com/files/onlinedocs/OPCLabs-OpcStudio/Latest/examples.html . Rem OPC client and subscriber examples in VBScript on GitHub: https://github.com/OPCLabs/Examples-QuickOPC-VBScript . Rem Missing some example? Ask us for it on our Online Forums, https://www.opclabs.com/forum/index ! You do not have to own Rem a commercial license in order to use Online Forums, and we reply to every post. Option Explicit ' Instantiate the client object and hook events Dim Client: Set Client = CreateObject("OpcLabs.EasyOpc.UA.EasyUAClient") WScript.ConnectObject Client, "Client_" WScript.Echo "Subscribing..." Dim MonitoringParameters: Set MonitoringParameters = CreateObject("OpcLabs.EasyOpc.UA.UAMonitoringParameters") MonitoringParameters.SamplingInterval = 1000 Dim MonitoredItemArguments1: Set MonitoredItemArguments1 = CreateObject("OpcLabs.EasyOpc.UA.OperationModel.EasyUAMonitoredItemArguments") MonitoredItemArguments1.EndpointDescriptor.UrlString = "opc.tcp://opcua.demo-this.com:51210/UA/SampleServer" MonitoredItemArguments1.NodeDescriptor.NodeId.ExpandedText = "nsu=http://test.org/UA/Data/ ;i=10845" MonitoredItemArguments1.MonitoringParameters = MonitoringParameters Dim MonitoredItemArguments2: Set MonitoredItemArguments2 = CreateObject("OpcLabs.EasyOpc.UA.OperationModel.EasyUAMonitoredItemArguments") MonitoredItemArguments2.EndpointDescriptor.UrlString = "opc.tcp://opcua.demo-this.com:51210/UA/SampleServer" MonitoredItemArguments2.NodeDescriptor.NodeId.ExpandedText = "nsu=http://test.org/UA/Data/ ;i=10853" MonitoredItemArguments2.MonitoringParameters = MonitoringParameters Dim MonitoredItemArguments3: Set MonitoredItemArguments3 = CreateObject("OpcLabs.EasyOpc.UA.OperationModel.EasyUAMonitoredItemArguments") MonitoredItemArguments3.EndpointDescriptor.UrlString = "opc.tcp://opcua.demo-this.com:51210/UA/SampleServer" MonitoredItemArguments3.NodeDescriptor.NodeId.ExpandedText = "nsu=http://test.org/UA/Data/ ;i=10855" MonitoredItemArguments3.MonitoringParameters = MonitoringParameters Dim arguments(2) Set arguments(0) = MonitoredItemArguments1 Set arguments(1) = MonitoredItemArguments2 Set arguments(2) = MonitoredItemArguments3 Dim handleArray: handleArray = Client.SubscribeMultipleMonitoredItems(arguments) Dim i: For i = LBound(handleArray) To UBound(handleArray) WScript.Echo "handleArray(" & i & "): " & handleArray(i) Next WScript.Echo "Processing monitored item changed events for 10 seconds..." WScript.Sleep 10 * 1000 WScript.Echo "Unsubscribing..." Client.UnsubscribeAllMonitoredItems WScript.Echo "Waiting for 5 seconds..." WScript.Sleep 5 * 1000 Sub Client_DataChangeNotification(Sender, e) ' Display the data Dim display: If e.Exception Is Nothing Then display = e.AttributeData Else display = e.ErrorMessageBrief WScript.Echo e.Arguments.NodeDescriptor & ":" & display End Sub
// This example shows how to subscribe to changes of multiple monitored items // and display each change, identifying the different subscriptions by an // integer. // // Find all latest examples here: https://opclabs.doc-that.com/files/onlinedocs/OPCLabs-OpcStudio/Latest/examples.html . // OPC client and subscriber examples in Object Pascal (Delphi) on GitHub: https://github.com/OPCLabs/Examples-QuickOPC-OP . // Missing some example? Ask us for it on our Online Forums, https://www.opclabs.com/forum/index ! You do not have to own // a commercial license in order to use Online Forums, and we reply to every post. type TIntegerClientEventHandlers124 = class procedure OnDataChangeNotification( ASender: TObject; sender: OleVariant; const eventArgs: _EasyUADataChangeNotificationEventArgs); end; procedure TIntegerClientEventHandlers124.OnDataChangeNotification( ASender: TObject; sender: OleVariant; const eventArgs: _EasyUADataChangeNotificationEventArgs); var stateAsInteger: Integer; begin // Obtain the integer state we have passed in. stateAsInteger := eventArgs.Arguments.State; if eventArgs.Succeeded then WriteLn(stateAsInteger, ': ', eventArgs.AttributeData.ToString) else WriteLn(stateAsInteger, ' *** Failure: ', eventArgs.ErrorMessageBrief); end; class procedure SubscribeMultipleMonitoredItems.StateAsInteger; var Arguments: OleVariant; Client: TEasyUAClient; ClientEventHandlers: TIntegerClientEventHandlers124; Handle: Cardinal; HandleArray: OleVariant; I: Cardinal; MonitoredItemArguments1, MonitoredItemArguments2, MonitoredItemArguments3: _EasyUAMonitoredItemArguments; MonitoringParameters: _UAMonitoringParameters; begin // Instantiate the client object and hook events Client := TEasyUAClient.Create(nil); ClientEventHandlers := TIntegerClientEventHandlers124.Create; Client.OnDataChangeNotification := ClientEventHandlers.OnDataChangeNotification; WriteLn('Subscribing...'); MonitoringParameters := CoUAMonitoringParameters.Create; MonitoringParameters.SamplingInterval := 1000; MonitoredItemArguments1 := CoEasyUAMonitoredItemArguments.Create; MonitoredItemArguments1.EndpointDescriptor.UrlString := //'http://opcua.demo-this.com:51211/UA/SampleServer'; //'https://opcua.demo-this.com:51212/UA/SampleServer/'; 'opc.tcp://opcua.demo-this.com:51210/UA/SampleServer'; MonitoredItemArguments1.NodeDescriptor.NodeId.ExpandedText := 'nsu=http://test.org/UA/Data/ ;i=10845'; MonitoredItemArguments1.MonitoringParameters := MonitoringParameters; MonitoredItemArguments1.SetState(1); // An integer we have chosen to identify the subscription MonitoredItemArguments2 := CoEasyUAMonitoredItemArguments.Create; MonitoredItemArguments2.EndpointDescriptor.UrlString := //'http://opcua.demo-this.com:51211/UA/SampleServer'; //'https://opcua.demo-this.com:51212/UA/SampleServer/'; 'opc.tcp://opcua.demo-this.com:51210/UA/SampleServer'; MonitoredItemArguments2.NodeDescriptor.NodeId.ExpandedText := 'nsu=http://test.org/UA/Data/ ;i=10853'; MonitoredItemArguments2.MonitoringParameters := MonitoringParameters; MonitoredItemArguments2.SetState(2); // An integer we have chosen to identify the subscription MonitoredItemArguments3 := CoEasyUAMonitoredItemArguments.Create; MonitoredItemArguments3.EndpointDescriptor.UrlString := //'http://opcua.demo-this.com:51211/UA/SampleServer'; //'https://opcua.demo-this.com:51212/UA/SampleServer/'; 'opc.tcp://opcua.demo-this.com:51210/UA/SampleServer'; MonitoredItemArguments3.NodeDescriptor.NodeId.ExpandedText := 'nsu=http://test.org/UA/Data/ ;i=10855'; MonitoredItemArguments3.MonitoringParameters := MonitoringParameters; MonitoredItemArguments3.SetState(3); // An integer we have chosen to identify the subscription Arguments := VarArrayCreate([0, 2], varVariant); Arguments[0] := MonitoredItemArguments1; Arguments[1] := MonitoredItemArguments2; Arguments[2] := MonitoredItemArguments3; TVarData(HandleArray).VType := varArray or varVariant; TVarData(HandleArray).VArray := PVarArray( Client.SubscribeMultipleMonitoredItems(Arguments)); for I := VarArrayLowBound(HandleArray, 1) to VarArrayHighBound(HandleArray, 1) do begin Handle := Cardinal(HandleArray[I]); WriteLn('HandleArray[', I, ']: ', Handle); end; WriteLn('Processing monitored item changed events for 10 seconds...'); PumpSleep(10*1000); WriteLn('Unsubscribing...'); Client.UnsubscribeAllMonitoredItems; WriteLn('Waiting for 5 seconds...'); Sleep(5*1000); WriteLn('Finished.'); VarClear(HandleArray); VarClear(Arguments); FreeAndNil(Client); FreeAndNil(ClientEventHandlers); end;
// This example shows how to subscribe to changes of multiple monitored items // and display each change, identifying the different subscriptions by an // integer. // // Find all latest examples here: https://opclabs.doc-that.com/files/onlinedocs/OPCLabs-OpcStudio/Latest/examples.html . // OPC client and subscriber examples in PHP on GitHub: https://github.com/OPCLabs/Examples-QuickOPC-PHP . // Missing some example? Ask us for it on our Online Forums, https://www.opclabs.com/forum/index ! You do not have to own // a commercial license in order to use Online Forums, and we reply to every post. class ClientEvents { function DataChangeNotification($Sender, $E) { // Obtain the integer state we have passed in. $stateAsInteger = $E->Arguments->State; if ($E->Succeeded) printf(" s\n", $stateAsInteger, $E->AttributeData); else printf("d *** Failures\n", $stateAsInteger, $E->ErrorMessageBrief); } } // Instantiate the client object and hook events $Client = new COM("OpcLabs.EasyOpc.UA.EasyUAClient"); $ClientEvents = new ClientEvents(); com_event_sink($Client, $ClientEvents, "DEasyUAClientEvents"); printf("Subscribing...\n"); $MonitoringParameters = new COM("OpcLabs.EasyOpc.UA.UAMonitoringParameters"); $MonitoringParameters->SamplingInterval = 1000; $MonitoredItemArguments1 = new COM("OpcLabs.EasyOpc.UA.OperationModel.EasyUAMonitoredItemArguments"); $MonitoredItemArguments1->EndpointDescriptor->UrlString = //"http://opcua.demo-this.com:51211/UA/SampleServer"; //"https://opcua.demo-this.com:51212/UA/SampleServer/"; "opc.tcp://opcua.demo-this.com:51210/UA/SampleServer"; $MonitoredItemArguments1->NodeDescriptor->NodeId->ExpandedText = "nsu=http://test.org/UA/Data/ ;i=10845"; $MonitoredItemArguments1->MonitoringParameters = $MonitoringParameters; $MonitoredItemArguments1->SetState(1); // An integer we have chosen to identify the subscription $MonitoredItemArguments2 = new COM("OpcLabs.EasyOpc.UA.OperationModel.EasyUAMonitoredItemArguments"); $MonitoredItemArguments2->EndpointDescriptor->UrlString = //"http://opcua.demo-this.com:51211/UA/SampleServer"; //"https://opcua.demo-this.com:51212/UA/SampleServer/"; "opc.tcp://opcua.demo-this.com:51210/UA/SampleServer"; $MonitoredItemArguments2->NodeDescriptor->NodeId->ExpandedText = "nsu=http://test.org/UA/Data/ ;i=10853"; $MonitoredItemArguments2->MonitoringParameters = $MonitoringParameters; $MonitoredItemArguments2->SetState(2); // An integer we have chosen to identify the subscription $MonitoredItemArguments3 = new COM("OpcLabs.EasyOpc.UA.OperationModel.EasyUAMonitoredItemArguments"); $MonitoredItemArguments3->EndpointDescriptor->UrlString = //"http://opcua.demo-this.com:51211/UA/SampleServer"; //"https://opcua.demo-this.com:51212/UA/SampleServer/"; "opc.tcp://opcua.demo-this.com:51210/UA/SampleServer"; $MonitoredItemArguments3->NodeDescriptor->NodeId->ExpandedText = "nsu=http://test.org/UA/Data/ ;i=10855"; $MonitoredItemArguments3->MonitoringParameters = $MonitoringParameters; $MonitoredItemArguments3->SetState(3); // An integer we have chosen to identify the subscription $arguments[0] = $MonitoredItemArguments1; $arguments[1] = $MonitoredItemArguments2; $arguments[2] = $MonitoredItemArguments3; $handleArray = $Client->SubscribeMultipleMonitoredItems($arguments); for ($i = 0; $i < count($handleArray); $i++) { printf("handleArray[d]d\n", $i, $handleArray[$i]); } printf("Processing monitored item changed events for 10 seconds...\n"); $startTime = time(); do { com_message_pump(1000); } while (time() < $startTime + 10); printf("Unsubscribing...\n"); $Client->UnsubscribeAllMonitoredItems; printf("Waiting for 5 seconds...\n"); $startTime = time(); do { com_message_pump(1000); } while (time() < $startTime + 5);
REM This example shows how to subscribe to changes of multiple monitored items REM and display each change, identifying the different subscriptions by an REM integer. REM REM Find all latest examples here: https://opclabs.doc-that.com/files/onlinedocs/OPCLabs-OpcStudio/Latest/examples.html . REM OPC client and subscriber examples in Visual Basic on GitHub: https://github.com/OPCLabs/Examples-QuickOPC-VB . REM Missing some example? Ask us for it on our Online Forums, https://www.opclabs.com/forum/index ! You do not have to own REM a commercial license in order to use Online Forums, and we reply to every post. ' The client object, with events 'Public WithEvents Client6 As EasyUAClient Public Sub SubscribeMultipleMonitoredItems_StateAsInteger_Command_Click() OutputText = "" Set Client6 = New EasyUAClient OutputText = OutputText & "Subscribing..." & vbCrLf Dim MonitoringParameters As New UAMonitoringParameters MonitoringParameters.SamplingInterval = 1000 Dim MonitoredItemArguments1 As New EasyUAMonitoredItemArguments MonitoredItemArguments1.endpointDescriptor.UrlString = "opc.tcp://opcua.demo-this.com:51210/UA/SampleServer" MonitoredItemArguments1.nodeDescriptor.NodeId.expandedText = "nsu=http://test.org/UA/Data/ ;i=10845" Set MonitoredItemArguments1.MonitoringParameters = MonitoringParameters MonitoredItemArguments1.SetState 1 ' An integer we have chosen to identify the subscription Dim MonitoredItemArguments2 As New EasyUAMonitoredItemArguments MonitoredItemArguments2.endpointDescriptor.UrlString = "opc.tcp://opcua.demo-this.com:51210/UA/SampleServer" MonitoredItemArguments2.nodeDescriptor.NodeId.expandedText = "nsu=http://test.org/UA/Data/ ;i=10853" Set MonitoredItemArguments2.MonitoringParameters = MonitoringParameters MonitoredItemArguments2.SetState 2 ' An integer we have chosen to identify the subscription Dim MonitoredItemArguments3 As New EasyUAMonitoredItemArguments MonitoredItemArguments3.endpointDescriptor.UrlString = "opc.tcp://opcua.demo-this.com:51210/UA/SampleServer" MonitoredItemArguments3.nodeDescriptor.NodeId.expandedText = "nsu=http://test.org/UA/Data/ ;i=10855" Set MonitoredItemArguments3.MonitoringParameters = MonitoringParameters MonitoredItemArguments3.SetState 3 ' An integer we have chosen to identify the subscription Dim arguments(2) As Variant Set arguments(0) = MonitoredItemArguments1 Set arguments(1) = MonitoredItemArguments2 Set arguments(2) = MonitoredItemArguments3 Dim handleArray As Variant handleArray = Client6.SubscribeMultipleMonitoredItems(arguments) Dim i As Long: For i = LBound(handleArray) To UBound(handleArray) OutputText = OutputText & "handleArray(" & i & "): " & handleArray(i) & vbCrLf Next OutputText = OutputText & "Processing monitored item changed events for 10 seconds..." & vbCrLf Pause 10000 OutputText = OutputText & "Unsubscribing..." & vbCrLf Call Client6.UnsubscribeAllMonitoredItems OutputText = OutputText & "Waiting for 5 seconds..." & vbCrLf Pause 5000 Set Client2 = Nothing OutputText = OutputText & "Finished." & vbCrLf End Sub Public Sub Client6_DataChangeNotification(ByVal sender As Variant, ByVal eventArgs As EasyUADataChangeNotificationEventArgs) ' Obtain the integer state we have passed in. Dim stateAsInteger As Integer: stateAsInteger = eventArgs.arguments.State If eventArgs.Succeeded Then OutputText = OutputText & stateAsInteger & ": " & eventArgs.AttributeData & vbCrLf Else OutputText = OutputText & stateAsInteger & " *** Failure: " & eventArgs.ErrorMessageBrief & vbCrLf End If End Sub
// This example shows how to subscribe to changes of multiple monitored items // and display each change, identifying the different subscriptions by an // object. // // Find all latest examples here: https://opclabs.doc-that.com/files/onlinedocs/OPCLabs-OpcStudio/Latest/examples.html . // OPC client and subscriber examples in Object Pascal (Delphi) on GitHub: https://github.com/OPCLabs/Examples-QuickOPC-OP . // Missing some example? Ask us for it on our Online Forums, https://www.opclabs.com/forum/index ! You do not have to own // a commercial license in order to use Online Forums, and we reply to every post. type TCustomObject = class Name: string; constructor Create(name: string); end; constructor TCustomObject.Create(name: string); begin Self.Name := name; end; type TObjectClientEventHandlers125 = class procedure OnDataChangeNotification( ASender: TObject; sender: OleVariant; const eventArgs: _EasyUADataChangeNotificationEventArgs); end; procedure TObjectClientEventHandlers125.OnDataChangeNotification( ASender: TObject; sender: OleVariant; const eventArgs: _EasyUADataChangeNotificationEventArgs); var stateAsObject: TCustomObject; begin // Obtain the custom object we have passed in. stateAsObject := TCustomObject(INT_PTR(eventArgs.Arguments.State)); // Display the data if eventArgs.Succeeded then WriteLn(stateAsObject.Name, ': ', eventArgs.AttributeData.ToString) else WriteLn(stateAsObject.Name, ' *** Failure: ', eventArgs.ErrorMessageBrief); end; class procedure SubscribeMultipleMonitoredItems.StateAsObject; var Arguments: OleVariant; Client: TEasyUAClient; ClientEventHandlers: TObjectClientEventHandlers125; Handle: Cardinal; HandleArray: OleVariant; I: Cardinal; MonitoredItemArguments1, MonitoredItemArguments2, MonitoredItemArguments3: _EasyUAMonitoredItemArguments; MonitoringParameters: _UAMonitoringParameters; CustomObject1, CustomObject2, CustomObject3: TCustomObject; begin CustomObject1 := TCustomObject.Create('First'); CustomObject2 := TCustomObject.Create('Second'); CustomObject3 := TCustomObject.Create('Third'); // Instantiate the client object and hook events Client := TEasyUAClient.Create(nil); ClientEventHandlers := TObjectClientEventHandlers125.Create; Client.OnDataChangeNotification := ClientEventHandlers.OnDataChangeNotification; WriteLn('Subscribing...'); MonitoringParameters := CoUAMonitoringParameters.Create; MonitoringParameters.SamplingInterval := 1000; MonitoredItemArguments1 := CoEasyUAMonitoredItemArguments.Create; MonitoredItemArguments1.EndpointDescriptor.UrlString := //'http://opcua.demo-this.com:51211/UA/SampleServer'; //'https://opcua.demo-this.com:51212/UA/SampleServer/'; 'opc.tcp://opcua.demo-this.com:51210/UA/SampleServer'; MonitoredItemArguments1.NodeDescriptor.NodeId.ExpandedText := 'nsu=http://test.org/UA/Data/ ;i=10845'; MonitoredItemArguments1.MonitoringParameters := MonitoringParameters; MonitoredItemArguments1.SetState(INT_PTR(CustomObject1)); // A custom object that corresponds to the subscription MonitoredItemArguments2 := CoEasyUAMonitoredItemArguments.Create; MonitoredItemArguments2.EndpointDescriptor.UrlString := //'http://opcua.demo-this.com:51211/UA/SampleServer'; //'https://opcua.demo-this.com:51212/UA/SampleServer/'; 'opc.tcp://opcua.demo-this.com:51210/UA/SampleServer'; MonitoredItemArguments2.NodeDescriptor.NodeId.ExpandedText := 'nsu=http://test.org/UA/Data/ ;i=10853'; MonitoredItemArguments2.MonitoringParameters := MonitoringParameters; MonitoredItemArguments2.SetState(INT_PTR(CustomObject2)); // A custom object that corresponds to the subscription MonitoredItemArguments3 := CoEasyUAMonitoredItemArguments.Create; MonitoredItemArguments3.EndpointDescriptor.UrlString := //'http://opcua.demo-this.com:51211/UA/SampleServer'; //'https://opcua.demo-this.com:51212/UA/SampleServer/'; 'opc.tcp://opcua.demo-this.com:51210/UA/SampleServer'; MonitoredItemArguments3.NodeDescriptor.NodeId.ExpandedText := 'nsu=http://test.org/UA/Data/ ;i=10855'; MonitoredItemArguments3.MonitoringParameters := MonitoringParameters; MonitoredItemArguments3.SetState(INT_PTR(CustomObject3)); // A custom object that corresponds to the subscription Arguments := VarArrayCreate([0, 2], varVariant); Arguments[0] := MonitoredItemArguments1; Arguments[1] := MonitoredItemArguments2; Arguments[2] := MonitoredItemArguments3; TVarData(HandleArray).VType := varArray or varVariant; TVarData(HandleArray).VArray := PVarArray( Client.SubscribeMultipleMonitoredItems(Arguments)); for I := VarArrayLowBound(HandleArray, 1) to VarArrayHighBound(HandleArray, 1) do begin Handle := Cardinal(HandleArray[I]); WriteLn('HandleArray[', I, ']: ', Handle); end; WriteLn('Processing monitored item changed events for 10 seconds...'); PumpSleep(10*1000); WriteLn('Unsubscribing...'); Client.UnsubscribeAllMonitoredItems; WriteLn('Waiting for 5 seconds...'); Sleep(5*1000); WriteLn('Finished.'); VarClear(HandleArray); VarClear(Arguments); FreeAndNil(Client); FreeAndNil(ClientEventHandlers); FreeAndNil(CustomObject1); FreeAndNil(CustomObject2); FreeAndNil(CustomObject3); end;