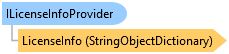
'Declaration
<CLSCompliantAttribute(True)> <ComVisibleAttribute(False)> <InterfaceCompositorAttribute(OpcLabs.BaseLib.Composition.LicenseInfoProviderCompositor)> Public Interface ILicenseInfoProvider
'Usage
Dim instance As ILicenseInfoProvider
[CLSCompliant(true)] [ComVisible(false)] [InterfaceCompositor(OpcLabs.BaseLib.Composition.LicenseInfoProviderCompositor)] public interface ILicenseInfoProvider
[CLSCompliant(true)] [ComVisible(false)] [InterfaceCompositor(OpcLabs.BaseLib.Composition.LicenseInfoProviderCompositor)] public interface class ILicenseInfoProvider
// Shows how to obtain the serial number of the active license, and determine whether it is a stock demo or trial license. // // Find all latest examples here: https://opclabs.doc-that.com/files/onlinedocs/OPCLabs-OpcStudio/Latest/examples.html . // OPC client and subscriber examples in C# on GitHub: https://github.com/OPCLabs/Examples-QuickOPC-CSharp . // Missing some example? Ask us for it on our Online Forums, https://www.opclabs.com/forum/index ! You do not have to own // a commercial license in order to use Online Forums, and we reply to every post. using System; using OpcLabs.EasyOpc.UA; namespace UADocExamples.Licensing { partial class LicenseInfo { public static void SerialNumber() { // Instantiate the client object. var client = new EasyUAClient(); // Obtain the serial number from the license info. long serialNumber = (uint)client.LicenseInfo["Multipurpose.SerialNumber"]; // Display the serial number. Console.WriteLine("SerialNumber: {0}", serialNumber); // Determine whether we are running as demo or trial. if ((1111110000 <= serialNumber) && (serialNumber <= 1111119999)) Console.WriteLine("This is a stock demo or trial license."); else Console.WriteLine("This is not a stock demo or trial license."); } } }
' Shows how to obtain the serial number of the active license, and determine whether it is a stock demo or trial license. ' ' Find all latest examples here: https://opclabs.doc-that.com/files/onlinedocs/OPCLabs-OpcStudio/Latest/examples.html . ' OPC client and subscriber examples in VB.NET on GitHub: https://github.com/OPCLabs/Examples-QuickOPC-VBNET . ' Missing some example? Ask us for it on our Online Forums, https://www.opclabs.com/forum/index ! You do not have to own ' a commercial license in order to use Online Forums, and we reply to every post. Imports OpcLabs.EasyOpc.UA Namespace Licensing Friend Class LicenseInfo Public Shared Sub SerialNumber() ' Instantiate the client object. Dim client = New EasyUAClient() ' Obtain the serial number from the license info. Dim serialNumber As Long = CUInt(client.LicenseInfo("Multipurpose.SerialNumber")) ' Display the serial number. Console.WriteLine("SerialNumber: {0}", serialNumber) ' Determine whether we are running as demo or trial. If (1111110000 <= serialNumber) And (serialNumber <= 1111119999) Then Console.WriteLine("This is a stock demo or trial license.") Else Console.WriteLine("This is not a stock demo or trial license.") End If End Sub End Class End Namespace
// Shows how to obtain the serial number of the active license, and determine whether it is a stock demo or trial license. // // Find all latest examples here: https://opclabs.doc-that.com/files/onlinedocs/OPCLabs-OpcStudio/Latest/examples.html . // OPC client and subscriber examples in Object Pascal (Delphi) on GitHub: https://github.com/OPCLabs/Examples-QuickOPC-OP . // Missing some example? Ask us for it on our Online Forums, https://www.opclabs.com/forum/index ! You do not have to own // a commercial license in order to use Online Forums, and we reply to every post. class procedure LicenseInfo.SerialNumber; var Client: _EasyUAClient; SerialNumber: Int64; begin // Instantiate the client object Client := CoEasyUAClient.Create; // Obtain the serial number from the license info. SerialNumber := Int64(client.LicenseInfo['Multipurpose.SerialNumber']); // Display the serial number. WriteLn('SerialNumber: ', SerialNumber); // Determine whether we are running as demo or trial. if ((1111110000 <= SerialNumber) and (SerialNumber <= 1111119999)) then WriteLn('This is a stock demo or trial license.') else WriteLn('This is not a stock demo or trial license.'); end;
// This example shows how to obtain nodes under a given node of the OPC-UA address space. // Shows how to obtain the serial number of the active license, and determine whether it is a stock demo or trial license. // // Find all latest examples here: https://opclabs.doc-that.com/files/onlinedocs/OPCLabs-OpcStudio/Latest/examples.html . // OPC client and subscriber examples in PHP on GitHub: https://github.com/OPCLabs/Examples-QuickOPC-PHP . // Missing some example? Ask us for it on our Online Forums, https://www.opclabs.com/forum/index ! You do not have to own // a commercial license in order to use Online Forums, and we reply to every post. // Instantiate the client object $Client = new COM("OpcLabs.EasyOpc.UA.EasyUAClient"); // Obtain the serial number from the license info. $SerialNumber = $Client->LicenseInfo["Multipurpose.SerialNumber"]; // Display the serial number. printf("SerialNumber: %s\n", $SerialNumber); // Determine whether we are running as demo or trial. if ((1111110000 <= $SerialNumber) and ($SerialNumber <= 1111119999)) printf("This is a stock demo or trial license.\n"); else printf("This is not a stock demo or trial license.\n");
REM Shows how to obtain the serial number of the active license, and determine whether it is a stock demo or trial license. REM REM Find all latest examples here: https://opclabs.doc-that.com/files/onlinedocs/OPCLabs-OpcStudio/Latest/examples.html . REM OPC client and subscriber examples in Visual Basic on GitHub: https://github.com/OPCLabs/Examples-QuickOPC-VB . REM Missing some example? Ask us for it on our Online Forums, https://www.opclabs.com/forum/index ! You do not have to own REM a commercial license in order to use Online Forums, and we reply to every post. Private Sub LicenseInfo_SerialNumber_Command_Click() OutputText = "" ' Instantiate the client object Dim client As New EasyUAClient ' Obtain the serial number from the license info. Dim serialNumber As Long serialNumber = client.LicenseInfo("Multipurpose.SerialNumber") ' Display the serial number. OutputText = OutputText & "Serial number: " & serialNumber & vbCrLf ' Determine whether we are running as demo or trial. If (1111110000 <= serialNumber) And (serialNumber <= 1111119999) Then OutputText = OutputText & "This is a stock demo or trial license." & vbCrLf Else OutputText = OutputText & "This is not a stock demo or trial license." & vbCrLf End If End Sub
# Shows how to obtain the serial number of the active license, and determine whether it is a stock demo or trial license. # # Find all latest examples here: https://opclabs.doc-that.com/files/onlinedocs/OPCLabs-OpcStudio/Latest/examples.html . # OPC client and subscriber examples in Python on GitHub: https://github.com/OPCLabs/Examples-QuickOPC-Python . # Missing some example? Ask us for it on our Online Forums, https://www.opclabs.com/forum/index ! You do not have to own # a commercial license in order to use Online Forums, and we reply to every post. # The QuickOPC package is needed. Install it using "pip install opclabs_quickopc". import opclabs_quickopc # Import .NET namespaces. from OpcLabs.EasyOpc.UA import * # Instantiate the client object. client = EasyUAClient() # Obtain the serial number from the license info. serialNumber = client.LicenseInfo.get_Item('Multipurpose.SerialNumber') # Display the serial number. print('SerialNumber: ', serialNumber, sep='') # Determine whether we are running as demo or trial. if (1111110000 <= serialNumber) and (serialNumber <= 1111119999): print('This is a stock demo or trial license.') else: print('This is not a stock demo or trial license.') print() print('Finished.')
// Shows how to obtain the serial number of the active license, and determine whether it is a stock demo or trial license. // You can use any OPC UA client, including our Connectivity Explorer and OpcCmd utility, to connect to the server. // // Find all latest examples here: https://opclabs.doc-that.com/files/onlinedocs/OPCLabs-OpcStudio/Latest/examples.html . // OPC client, server and subscriber examples in C# on GitHub: https://github.com/OPCLabs/Examples-OPCStudio-CSharp . // Missing some example? Ask us for it on our Online Forums, https://www.opclabs.com/forum/index ! You do not have to own // a commercial license in order to use Online Forums, and we reply to every post. using System; using OpcLabs.EasyOpc.UA; namespace UAServerDocExamples.Licensing { partial class LicenseInfo { public static void SerialNumber() { // Instantiate the server object. var server = new EasyUAServer(); // Obtain the serial number from the license info. long serialNumber = (uint)server.LicenseInfo["Multipurpose.SerialNumber"]; // Display the serial number. Console.WriteLine("SerialNumber: {0}", serialNumber); // Determine whether we are running as demo or trial. if ((1111110000 <= serialNumber) && (serialNumber <= 1111119999)) Console.WriteLine("This is a stock demo or trial license."); else Console.WriteLine("This is not a stock demo or trial license."); } } }
' Shows how to obtain the serial number of the active license, and determine whether it is a stock demo or trial license. ' You can use any OPC UA client, including our Connectivity Explorer and OpcCmd utility, to connect to the server. ' ' Find all latest examples here: https://opclabs.doc-that.com/files/onlinedocs/OPCLabs-OpcStudio/Latest/examples.html . ' OPC client and subscriber examples in VB.NET on GitHub: https://github.com/OPCLabs/Examples-QuickOPC-VBNET . ' Missing some example? Ask us for it on our Online Forums, https://www.opclabs.com/forum/index ! You do not have to own ' a commercial license in order to use Online Forums, and we reply to every post. Imports OpcLabs.EasyOpc.UA Namespace Licensing Partial Friend Class LicenseInfo Shared Sub SerialNumber() ' Instantiate the server object. Dim server = New EasyUAServer() ' Obtain the serial number from the license info. Dim serialNumber As Long = CUInt(server.LicenseInfo("Multipurpose.SerialNumber")) ' Display the serial number. Console.WriteLine("SerialNumber: {0}", serialNumber) ' Determine whether we are running as demo or trial. If (1111110000 <= serialNumber) And (serialNumber <= 1111119999) Then Console.WriteLine("This is a stock demo or trial license.") Else Console.WriteLine("This is not a stock demo or trial license.") End If End Sub End Class End Namespace